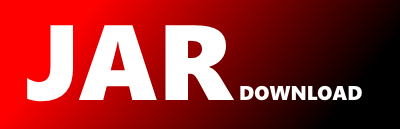
com.github.GBSEcom.model.Address Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification
* Payment Gateway API for payment processing.
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Address
*/
public class Address {
@SerializedName("company")
private String company = null;
@SerializedName("address1")
private String address1 = null;
@SerializedName("address2")
private String address2 = null;
@SerializedName("city")
private String city = null;
@SerializedName("region")
private String region = null;
@SerializedName("postalCode")
private String postalCode = null;
@SerializedName("country")
private String country = null;
public Address company(String company) {
this.company = company;
return this;
}
/**
* If it's a business, enter the name here.
* @return company
**/
@ApiModelProperty(value = "If it's a business, enter the name here.")
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
public Address address1(String address1) {
this.address1 = address1;
return this;
}
/**
* street address or P.O.Box number
* @return address1
**/
@ApiModelProperty(value = "street address or P.O.Box number")
public String getAddress1() {
return address1;
}
public void setAddress1(String address1) {
this.address1 = address1;
}
public Address address2(String address2) {
this.address2 = address2;
return this;
}
/**
* additional address information
* @return address2
**/
@ApiModelProperty(value = "additional address information")
public String getAddress2() {
return address2;
}
public void setAddress2(String address2) {
this.address2 = address2;
}
public Address city(String city) {
this.city = city;
return this;
}
/**
* City or Locality
* @return city
**/
@ApiModelProperty(value = "City or Locality")
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public Address region(String region) {
this.region = region;
return this;
}
/**
* State or Province
* @return region
**/
@ApiModelProperty(value = "State or Province")
public String getRegion() {
return region;
}
public void setRegion(String region) {
this.region = region;
}
public Address postalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
/**
* ZIP code or postal code
* @return postalCode
**/
@ApiModelProperty(value = "ZIP code or postal code")
public String getPostalCode() {
return postalCode;
}
public void setPostalCode(String postalCode) {
this.postalCode = postalCode;
}
public Address country(String country) {
this.country = country;
return this;
}
/**
* Country name
* @return country
**/
@ApiModelProperty(value = "Country name")
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Address address = (Address) o;
return Objects.equals(this.company, address.company) &&
Objects.equals(this.address1, address.address1) &&
Objects.equals(this.address2, address.address2) &&
Objects.equals(this.city, address.city) &&
Objects.equals(this.region, address.region) &&
Objects.equals(this.postalCode, address.postalCode) &&
Objects.equals(this.country, address.country);
}
@Override
public int hashCode() {
return Objects.hash(company, address1, address2, city, region, postalCode, country);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Address {\n");
sb.append(" company: ").append(toIndentedString(company)).append("\n");
sb.append(" address1: ").append(toIndentedString(address1)).append("\n");
sb.append(" address2: ").append(toIndentedString(address2)).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" region: ").append(toIndentedString(region)).append("\n");
sb.append(" postalCode: ").append(toIndentedString(postalCode)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy