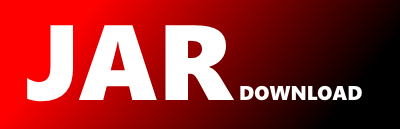
com.github.GBSEcom.model.AmountComponents Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification
* Payment Gateway API for payment processing.
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
/**
* AmountComponents
*/
public class AmountComponents {
@SerializedName("subtotal")
private BigDecimal subtotal = null;
@SerializedName("vatAmount")
private BigDecimal vatAmount = null;
@SerializedName("localTax")
private BigDecimal localTax = null;
@SerializedName("shipping")
private BigDecimal shipping = null;
@SerializedName("cashback")
private BigDecimal cashback = null;
@SerializedName("tip")
private BigDecimal tip = null;
@SerializedName("convenienceFee")
private BigDecimal convenienceFee = null;
public AmountComponents subtotal(BigDecimal subtotal) {
this.subtotal = subtotal;
return this;
}
/**
* Get subtotal
* @return subtotal
**/
@ApiModelProperty(example = "8.0", value = "")
public BigDecimal getSubtotal() {
return subtotal;
}
public void setSubtotal(BigDecimal subtotal) {
this.subtotal = subtotal;
}
public AmountComponents vatAmount(BigDecimal vatAmount) {
this.vatAmount = vatAmount;
return this;
}
/**
* Get vatAmount
* @return vatAmount
**/
@ApiModelProperty(example = "0.0", value = "")
public BigDecimal getVatAmount() {
return vatAmount;
}
public void setVatAmount(BigDecimal vatAmount) {
this.vatAmount = vatAmount;
}
public AmountComponents localTax(BigDecimal localTax) {
this.localTax = localTax;
return this;
}
/**
* Get localTax
* @return localTax
**/
@ApiModelProperty(example = "1.3", value = "")
public BigDecimal getLocalTax() {
return localTax;
}
public void setLocalTax(BigDecimal localTax) {
this.localTax = localTax;
}
public AmountComponents shipping(BigDecimal shipping) {
this.shipping = shipping;
return this;
}
/**
* Get shipping
* @return shipping
**/
@ApiModelProperty(example = "1.0", value = "")
public BigDecimal getShipping() {
return shipping;
}
public void setShipping(BigDecimal shipping) {
this.shipping = shipping;
}
public AmountComponents cashback(BigDecimal cashback) {
this.cashback = cashback;
return this;
}
/**
* Get cashback
* @return cashback
**/
@ApiModelProperty(example = "2.0", value = "")
public BigDecimal getCashback() {
return cashback;
}
public void setCashback(BigDecimal cashback) {
this.cashback = cashback;
}
public AmountComponents tip(BigDecimal tip) {
this.tip = tip;
return this;
}
/**
* Get tip
* @return tip
**/
@ApiModelProperty(example = "6.0", value = "")
public BigDecimal getTip() {
return tip;
}
public void setTip(BigDecimal tip) {
this.tip = tip;
}
public AmountComponents convenienceFee(BigDecimal convenienceFee) {
this.convenienceFee = convenienceFee;
return this;
}
/**
* Get convenienceFee
* @return convenienceFee
**/
@ApiModelProperty(example = "2.0", value = "")
public BigDecimal getConvenienceFee() {
return convenienceFee;
}
public void setConvenienceFee(BigDecimal convenienceFee) {
this.convenienceFee = convenienceFee;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AmountComponents amountComponents = (AmountComponents) o;
return Objects.equals(this.subtotal, amountComponents.subtotal) &&
Objects.equals(this.vatAmount, amountComponents.vatAmount) &&
Objects.equals(this.localTax, amountComponents.localTax) &&
Objects.equals(this.shipping, amountComponents.shipping) &&
Objects.equals(this.cashback, amountComponents.cashback) &&
Objects.equals(this.tip, amountComponents.tip) &&
Objects.equals(this.convenienceFee, amountComponents.convenienceFee);
}
@Override
public int hashCode() {
return Objects.hash(subtotal, vatAmount, localTax, shipping, cashback, tip, convenienceFee);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AmountComponents {\n");
sb.append(" subtotal: ").append(toIndentedString(subtotal)).append("\n");
sb.append(" vatAmount: ").append(toIndentedString(vatAmount)).append("\n");
sb.append(" localTax: ").append(toIndentedString(localTax)).append("\n");
sb.append(" shipping: ").append(toIndentedString(shipping)).append("\n");
sb.append(" cashback: ").append(toIndentedString(cashback)).append("\n");
sb.append(" tip: ").append(toIndentedString(tip)).append("\n");
sb.append(" convenienceFee: ").append(toIndentedString(convenienceFee)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy