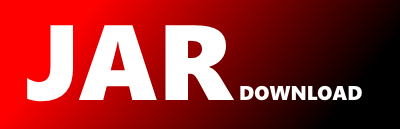
com.github.GBSEcom.model.InstallmentOptions Maven / Gradle / Ivy
/*
* Payment Gateway API Specification
* Payment Gateway API for payment processing.
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Indicates that the total sum payable is divided for payment at successive fixed times.
*/
@ApiModel(description = "Indicates that the total sum payable is divided for payment at successive fixed times.")
public class InstallmentOptions {
@SerializedName("numberOfInstallments")
private Integer numberOfInstallments = null;
@SerializedName("installmentsInterest")
private Boolean installmentsInterest = null;
@SerializedName("installmentDelayMonths")
private Integer installmentDelayMonths = null;
public InstallmentOptions numberOfInstallments(Integer numberOfInstallments) {
this.numberOfInstallments = numberOfInstallments;
return this;
}
/**
* Number of installments for a Sale transaction if the customer pays the total amount in multiple transactions
* minimum: 1
* maximum: 99
* @return numberOfInstallments
**/
@ApiModelProperty(value = "Number of installments for a Sale transaction if the customer pays the total amount in multiple transactions")
public Integer getNumberOfInstallments() {
return numberOfInstallments;
}
public void setNumberOfInstallments(Integer numberOfInstallments) {
this.numberOfInstallments = numberOfInstallments;
}
public InstallmentOptions installmentsInterest(Boolean installmentsInterest) {
this.installmentsInterest = installmentsInterest;
return this;
}
/**
* Indicates whether the installment interest amount has been applied. Possible values are \"yes\" or \"no\".
* @return installmentsInterest
**/
@ApiModelProperty(value = "Indicates whether the installment interest amount has been applied. Possible values are \"yes\" or \"no\".")
public Boolean isInstallmentsInterest() {
return installmentsInterest;
}
public void setInstallmentsInterest(Boolean installmentsInterest) {
this.installmentsInterest = installmentsInterest;
}
public InstallmentOptions installmentDelayMonths(Integer installmentDelayMonths) {
this.installmentDelayMonths = installmentDelayMonths;
return this;
}
/**
* The number of months the first installment payment will be delayed
* minimum: 1
* maximum: 99
* @return installmentDelayMonths
**/
@ApiModelProperty(value = "The number of months the first installment payment will be delayed")
public Integer getInstallmentDelayMonths() {
return installmentDelayMonths;
}
public void setInstallmentDelayMonths(Integer installmentDelayMonths) {
this.installmentDelayMonths = installmentDelayMonths;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
InstallmentOptions installmentOptions = (InstallmentOptions) o;
return Objects.equals(this.numberOfInstallments, installmentOptions.numberOfInstallments) &&
Objects.equals(this.installmentsInterest, installmentOptions.installmentsInterest) &&
Objects.equals(this.installmentDelayMonths, installmentOptions.installmentDelayMonths);
}
@Override
public int hashCode() {
return Objects.hash(numberOfInstallments, installmentsInterest, installmentDelayMonths);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class InstallmentOptions {\n");
sb.append(" numberOfInstallments: ").append(toIndentedString(numberOfInstallments)).append("\n");
sb.append(" installmentsInterest: ").append(toIndentedString(installmentsInterest)).append("\n");
sb.append(" installmentDelayMonths: ").append(toIndentedString(installmentDelayMonths)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy