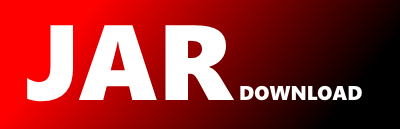
com.github.GBSEcom.model.PaymentSchedulesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification
* Payment Gateway API for payment processing.
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Response from the gateway scheduler creation call.
*/
@ApiModel(description = "Response from the gateway scheduler creation call.")
public class PaymentSchedulesResponse {
@SerializedName("clientRequestId")
private String clientRequestId = null;
@SerializedName("apiTraceId")
private String apiTraceId = null;
/**
* Result of requested operation. If it's anything other than 'SUCCESS', please refer to 400s HTTP error codes or decline. See Error object for details.
*/
@JsonAdapter(TransactionStatusEnum.Adapter.class)
public enum TransactionStatusEnum {
SUCCESS("SUCCESS"),
VALIDATION_FAILED("VALIDATION_FAILED"),
PROCESSING_FAILED("PROCESSING_FAILED"),
FAILURE("FAILURE");
private String value;
TransactionStatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TransactionStatusEnum fromValue(String text) {
for (TransactionStatusEnum b : TransactionStatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TransactionStatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TransactionStatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TransactionStatusEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("transactionStatus")
private TransactionStatusEnum transactionStatus = null;
@SerializedName("orderId")
private String orderId = null;
public PaymentSchedulesResponse clientRequestId(String clientRequestId) {
this.clientRequestId = clientRequestId;
return this;
}
/**
* Echoes back the value in the Request header for tracking.
* @return clientRequestId
**/
@ApiModelProperty(example = "30dd879c-ee2f-11db-8314-0800200c9a66", required = true, value = "Echoes back the value in the Request header for tracking.")
public String getClientRequestId() {
return clientRequestId;
}
public void setClientRequestId(String clientRequestId) {
this.clientRequestId = clientRequestId;
}
public PaymentSchedulesResponse apiTraceId(String apiTraceId) {
this.apiTraceId = apiTraceId;
return this;
}
/**
* Request identifier in API, can be used to request logs from the support.
* @return apiTraceId
**/
@ApiModelProperty(example = "1231234135", required = true, value = "Request identifier in API, can be used to request logs from the support.")
public String getApiTraceId() {
return apiTraceId;
}
public void setApiTraceId(String apiTraceId) {
this.apiTraceId = apiTraceId;
}
public PaymentSchedulesResponse transactionStatus(TransactionStatusEnum transactionStatus) {
this.transactionStatus = transactionStatus;
return this;
}
/**
* Result of requested operation. If it's anything other than 'SUCCESS', please refer to 400s HTTP error codes or decline. See Error object for details.
* @return transactionStatus
**/
@ApiModelProperty(example = "SUCCESS", required = true, value = "Result of requested operation. If it's anything other than 'SUCCESS', please refer to 400s HTTP error codes or decline. See Error object for details.")
public TransactionStatusEnum getTransactionStatus() {
return transactionStatus;
}
public void setTransactionStatus(TransactionStatusEnum transactionStatus) {
this.transactionStatus = transactionStatus;
}
public PaymentSchedulesResponse orderId(String orderId) {
this.orderId = orderId;
return this;
}
/**
* Client Order ID if supplied by client, otherwise the Order ID.
* @return orderId
**/
@ApiModelProperty(example = "123456", value = "Client Order ID if supplied by client, otherwise the Order ID.")
public String getOrderId() {
return orderId;
}
public void setOrderId(String orderId) {
this.orderId = orderId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentSchedulesResponse paymentSchedulesResponse = (PaymentSchedulesResponse) o;
return Objects.equals(this.clientRequestId, paymentSchedulesResponse.clientRequestId) &&
Objects.equals(this.apiTraceId, paymentSchedulesResponse.apiTraceId) &&
Objects.equals(this.transactionStatus, paymentSchedulesResponse.transactionStatus) &&
Objects.equals(this.orderId, paymentSchedulesResponse.orderId);
}
@Override
public int hashCode() {
return Objects.hash(clientRequestId, apiTraceId, transactionStatus, orderId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentSchedulesResponse {\n");
sb.append(" clientRequestId: ").append(toIndentedString(clientRequestId)).append("\n");
sb.append(" apiTraceId: ").append(toIndentedString(apiTraceId)).append("\n");
sb.append(" transactionStatus: ").append(toIndentedString(transactionStatus)).append("\n");
sb.append(" orderId: ").append(toIndentedString(orderId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy