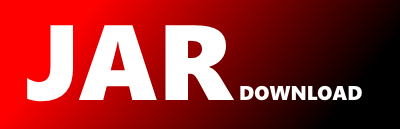
com.github.GBSEcom.model.PaymentUrlResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification
* Payment Gateway API for payment processing.
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Response from embedded payment link request.
*/
@ApiModel(description = "Response from embedded payment link request.")
public class PaymentUrlResponse {
@SerializedName("clientRequestId")
private String clientRequestId = null;
@SerializedName("apiTraceId")
private String apiTraceId = null;
/**
* Request status. If it's anything other than 'SUCCESS', please refer to 400s HTTP error codes or decline. See Error object for details.
*/
@JsonAdapter(RequestStatusEnum.Adapter.class)
public enum RequestStatusEnum {
SUCCESS("SUCCESS"),
VALIDATION_FAILED("VALIDATION_FAILED"),
PROCESSING_FAILED("PROCESSING_FAILED"),
FAILURE("FAILURE");
private String value;
RequestStatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static RequestStatusEnum fromValue(String text) {
for (RequestStatusEnum b : RequestStatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final RequestStatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public RequestStatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return RequestStatusEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("requestStatus")
private RequestStatusEnum requestStatus = null;
@SerializedName("orderId")
private String orderId = null;
@SerializedName("paymentUrl")
private String paymentUrl = null;
@SerializedName("transactionId")
private String transactionId = null;
public PaymentUrlResponse clientRequestId(String clientRequestId) {
this.clientRequestId = clientRequestId;
return this;
}
/**
* Echoes back the value in the Request header for tracking.
* @return clientRequestId
**/
@ApiModelProperty(example = "30dd879c-ee2f-11db-8314-0800200c9a66", required = true, value = "Echoes back the value in the Request header for tracking.")
public String getClientRequestId() {
return clientRequestId;
}
public void setClientRequestId(String clientRequestId) {
this.clientRequestId = clientRequestId;
}
public PaymentUrlResponse apiTraceId(String apiTraceId) {
this.apiTraceId = apiTraceId;
return this;
}
/**
* Request identifier in API, can be used to request logs from the support.
* @return apiTraceId
**/
@ApiModelProperty(example = "1231234135", required = true, value = "Request identifier in API, can be used to request logs from the support.")
public String getApiTraceId() {
return apiTraceId;
}
public void setApiTraceId(String apiTraceId) {
this.apiTraceId = apiTraceId;
}
public PaymentUrlResponse requestStatus(RequestStatusEnum requestStatus) {
this.requestStatus = requestStatus;
return this;
}
/**
* Request status. If it's anything other than 'SUCCESS', please refer to 400s HTTP error codes or decline. See Error object for details.
* @return requestStatus
**/
@ApiModelProperty(example = "SUCCESS", required = true, value = "Request status. If it's anything other than 'SUCCESS', please refer to 400s HTTP error codes or decline. See Error object for details.")
public RequestStatusEnum getRequestStatus() {
return requestStatus;
}
public void setRequestStatus(RequestStatusEnum requestStatus) {
this.requestStatus = requestStatus;
}
public PaymentUrlResponse orderId(String orderId) {
this.orderId = orderId;
return this;
}
/**
* Client Order ID if supplied by client, otherwise the Order ID.
* @return orderId
**/
@ApiModelProperty(example = "123456", value = "Client Order ID if supplied by client, otherwise the Order ID.")
public String getOrderId() {
return orderId;
}
public void setOrderId(String orderId) {
this.orderId = orderId;
}
public PaymentUrlResponse paymentUrl(String paymentUrl) {
this.paymentUrl = paymentUrl;
return this;
}
/**
* Get paymentUrl
* @return paymentUrl
**/
@ApiModelProperty(value = "")
public String getPaymentUrl() {
return paymentUrl;
}
public void setPaymentUrl(String paymentUrl) {
this.paymentUrl = paymentUrl;
}
public PaymentUrlResponse transactionId(String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* ID code from the transaction.
* @return transactionId
**/
@ApiModelProperty(example = "123978432", value = "ID code from the transaction.")
public String getTransactionId() {
return transactionId;
}
public void setTransactionId(String transactionId) {
this.transactionId = transactionId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentUrlResponse paymentUrlResponse = (PaymentUrlResponse) o;
return Objects.equals(this.clientRequestId, paymentUrlResponse.clientRequestId) &&
Objects.equals(this.apiTraceId, paymentUrlResponse.apiTraceId) &&
Objects.equals(this.requestStatus, paymentUrlResponse.requestStatus) &&
Objects.equals(this.orderId, paymentUrlResponse.orderId) &&
Objects.equals(this.paymentUrl, paymentUrlResponse.paymentUrl) &&
Objects.equals(this.transactionId, paymentUrlResponse.transactionId);
}
@Override
public int hashCode() {
return Objects.hash(clientRequestId, apiTraceId, requestStatus, orderId, paymentUrl, transactionId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentUrlResponse {\n");
sb.append(" clientRequestId: ").append(toIndentedString(clientRequestId)).append("\n");
sb.append(" apiTraceId: ").append(toIndentedString(apiTraceId)).append("\n");
sb.append(" requestStatus: ").append(toIndentedString(requestStatus)).append("\n");
sb.append(" orderId: ").append(toIndentedString(orderId)).append("\n");
sb.append(" paymentUrl: ").append(toIndentedString(paymentUrl)).append("\n");
sb.append(" transactionId: ").append(toIndentedString(transactionId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy