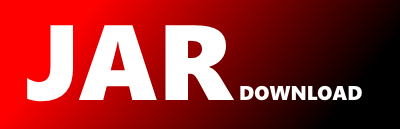
com.github.GBSEcom.model.Sepa Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification
* Payment Gateway API for payment processing.
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import com.github.GBSEcom.model.SepaMandate;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* The payment object for SEPA DD. The iban, billing_address.name and country elements are required for this payment type.
*/
@ApiModel(description = "The payment object for SEPA DD. The iban, billing_address.name and country elements are required for this payment type.")
public class Sepa {
@SerializedName("iban")
private String iban = null;
@SerializedName("name")
private String name = null;
@SerializedName("country")
private String country = null;
@SerializedName("email")
private String email = null;
@SerializedName("mandate")
private SepaMandate mandate = null;
public Sepa iban(String iban) {
this.iban = iban;
return this;
}
/**
* Bank account in IBAN format
* @return iban
**/
@ApiModelProperty(example = "DE34500100600032121604", required = true, value = "Bank account in IBAN format")
public String getIban() {
return iban;
}
public void setIban(String iban) {
this.iban = iban;
}
public Sepa name(String name) {
this.name = name;
return this;
}
/**
* The name of the payer
* @return name
**/
@ApiModelProperty(required = true, value = "The name of the payer")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Sepa country(String country) {
this.country = country;
return this;
}
/**
* Country of residence of the payer using the ISO 3166 standard (http://en.wikipedia.org/wiki/ISO_3166)
* @return country
**/
@ApiModelProperty(required = true, value = "Country of residence of the payer using the ISO 3166 standard (http://en.wikipedia.org/wiki/ISO_3166)")
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public Sepa email(String email) {
this.email = email;
return this;
}
/**
* The email address of the payer
* @return email
**/
@ApiModelProperty(value = "The email address of the payer")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Sepa mandate(SepaMandate mandate) {
this.mandate = mandate;
return this;
}
/**
* Get mandate
* @return mandate
**/
@ApiModelProperty(required = true, value = "")
public SepaMandate getMandate() {
return mandate;
}
public void setMandate(SepaMandate mandate) {
this.mandate = mandate;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Sepa sepa = (Sepa) o;
return Objects.equals(this.iban, sepa.iban) &&
Objects.equals(this.name, sepa.name) &&
Objects.equals(this.country, sepa.country) &&
Objects.equals(this.email, sepa.email) &&
Objects.equals(this.mandate, sepa.mandate);
}
@Override
public int hashCode() {
return Objects.hash(iban, name, country, email, mandate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Sepa {\n");
sb.append(" iban: ").append(toIndentedString(iban)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" mandate: ").append(toIndentedString(mandate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy