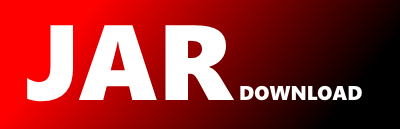
com.github.GBSEcom.model.StoredCredential Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification
* Payment Gateway API for payment processing.
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Object for sending visa store credential
*/
@ApiModel(description = "Object for sending visa store credential")
public class StoredCredential {
/**
* Indicates if the transaction is first or subsequent. Valid values are 'FIRST' and 'SUBSEQUENT'
*/
@JsonAdapter(SequenceEnum.Adapter.class)
public enum SequenceEnum {
FIRST("FIRST"),
SUBSEQUENT("SUBSEQUENT");
private String value;
SequenceEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static SequenceEnum fromValue(String text) {
for (SequenceEnum b : SequenceEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final SequenceEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public SequenceEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return SequenceEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("sequence")
private SequenceEnum sequence = null;
@SerializedName("scheduled")
private Boolean scheduled = null;
@SerializedName("referencedSchemeTransactionId")
private String referencedSchemeTransactionId = null;
/**
* Indicates whether it is a merchant initiated or explicitly consented by card holder. Valid values are 'MERCHANT' and 'CARDHOLDER'
*/
@JsonAdapter(InitiatorEnum.Adapter.class)
public enum InitiatorEnum {
MERCHANT("MERCHANT"),
CARDHOLDER("CARDHOLDER");
private String value;
InitiatorEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static InitiatorEnum fromValue(String text) {
for (InitiatorEnum b : InitiatorEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final InitiatorEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public InitiatorEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return InitiatorEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("initiator")
private InitiatorEnum initiator = null;
public StoredCredential sequence(SequenceEnum sequence) {
this.sequence = sequence;
return this;
}
/**
* Indicates if the transaction is first or subsequent. Valid values are 'FIRST' and 'SUBSEQUENT'
* @return sequence
**/
@ApiModelProperty(required = true, value = "Indicates if the transaction is first or subsequent. Valid values are 'FIRST' and 'SUBSEQUENT'")
public SequenceEnum getSequence() {
return sequence;
}
public void setSequence(SequenceEnum sequence) {
this.sequence = sequence;
}
public StoredCredential scheduled(Boolean scheduled) {
this.scheduled = scheduled;
return this;
}
/**
* Indicates if the transaction is scheduled or part of a installment
* @return scheduled
**/
@ApiModelProperty(required = true, value = "Indicates if the transaction is scheduled or part of a installment")
public Boolean isScheduled() {
return scheduled;
}
public void setScheduled(Boolean scheduled) {
this.scheduled = scheduled;
}
public StoredCredential referencedSchemeTransactionId(String referencedSchemeTransactionId) {
this.referencedSchemeTransactionId = referencedSchemeTransactionId;
return this;
}
/**
* The transaction id received from schemes for the initial transaction. Required if sequence is 'SUBSEQUENT'
* @return referencedSchemeTransactionId
**/
@ApiModelProperty(value = "The transaction id received from schemes for the initial transaction. Required if sequence is 'SUBSEQUENT'")
public String getReferencedSchemeTransactionId() {
return referencedSchemeTransactionId;
}
public void setReferencedSchemeTransactionId(String referencedSchemeTransactionId) {
this.referencedSchemeTransactionId = referencedSchemeTransactionId;
}
public StoredCredential initiator(InitiatorEnum initiator) {
this.initiator = initiator;
return this;
}
/**
* Indicates whether it is a merchant initiated or explicitly consented by card holder. Valid values are 'MERCHANT' and 'CARDHOLDER'
* @return initiator
**/
@ApiModelProperty(value = "Indicates whether it is a merchant initiated or explicitly consented by card holder. Valid values are 'MERCHANT' and 'CARDHOLDER'")
public InitiatorEnum getInitiator() {
return initiator;
}
public void setInitiator(InitiatorEnum initiator) {
this.initiator = initiator;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
StoredCredential storedCredential = (StoredCredential) o;
return Objects.equals(this.sequence, storedCredential.sequence) &&
Objects.equals(this.scheduled, storedCredential.scheduled) &&
Objects.equals(this.referencedSchemeTransactionId, storedCredential.referencedSchemeTransactionId) &&
Objects.equals(this.initiator, storedCredential.initiator);
}
@Override
public int hashCode() {
return Objects.hash(sequence, scheduled, referencedSchemeTransactionId, initiator);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class StoredCredential {\n");
sb.append(" sequence: ").append(toIndentedString(sequence)).append("\n");
sb.append(" scheduled: ").append(toIndentedString(scheduled)).append("\n");
sb.append(" referencedSchemeTransactionId: ").append(toIndentedString(referencedSchemeTransactionId)).append("\n");
sb.append(" initiator: ").append(toIndentedString(initiator)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy