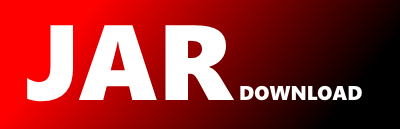
com.github.GBSEcom.model.AccessTokenResponse Maven / Gradle / Ivy
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Access token generation response.
*/
@ApiModel(description = "Access token generation response.")
public class AccessTokenResponse {
public static final String SERIALIZED_NAME_TOKEN_ID = "tokenId";
@SerializedName(SERIALIZED_NAME_TOKEN_ID)
private String tokenId;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private String status;
public static final String SERIALIZED_NAME_ISSUED_ON = "issuedOn";
@SerializedName(SERIALIZED_NAME_ISSUED_ON)
private String issuedOn;
public static final String SERIALIZED_NAME_EXPIRES_IN_SECONDS = "expiresInSeconds";
@SerializedName(SERIALIZED_NAME_EXPIRES_IN_SECONDS)
private String expiresInSeconds;
public static final String SERIALIZED_NAME_PUBLIC_KEY_BASE64 = "publicKeyBase64";
@SerializedName(SERIALIZED_NAME_PUBLIC_KEY_BASE64)
private String publicKeyBase64;
public static final String SERIALIZED_NAME_ALGORITHM = "algorithm";
@SerializedName(SERIALIZED_NAME_ALGORITHM)
private String algorithm;
public static final String SERIALIZED_NAME_CLIENT_REQUEST_ID = "clientRequestId";
@SerializedName(SERIALIZED_NAME_CLIENT_REQUEST_ID)
private String clientRequestId;
public AccessTokenResponse tokenId(String tokenId) {
this.tokenId = tokenId;
return this;
}
/**
* Access token for authentication.
* @return tokenId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "gliF92ypj9cKRWUP8lpRIbI3bhNf", value = "Access token for authentication.")
public String getTokenId() {
return tokenId;
}
public void setTokenId(String tokenId) {
this.tokenId = tokenId;
}
public AccessTokenResponse status(String status) {
this.status = status;
return this;
}
/**
* The token status.
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "ACTIVE", value = "The token status.")
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public AccessTokenResponse issuedOn(String issuedOn) {
this.issuedOn = issuedOn;
return this;
}
/**
* Access token issued time in milliseconds.
* @return issuedOn
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1579021570941", value = "Access token issued time in milliseconds.")
public String getIssuedOn() {
return issuedOn;
}
public void setIssuedOn(String issuedOn) {
this.issuedOn = issuedOn;
}
public AccessTokenResponse expiresInSeconds(String expiresInSeconds) {
this.expiresInSeconds = expiresInSeconds;
return this;
}
/**
* Access token expiration time.
* @return expiresInSeconds
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "899", value = "Access token expiration time.")
public String getExpiresInSeconds() {
return expiresInSeconds;
}
public void setExpiresInSeconds(String expiresInSeconds) {
this.expiresInSeconds = expiresInSeconds;
}
public AccessTokenResponse publicKeyBase64(String publicKeyBase64) {
this.publicKeyBase64 = publicKeyBase64;
return this;
}
/**
* Public key to encrypt data.
* @return publicKeyBase64
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "LS0tLS1CRUdJTiBQLbnFSNXRnVmc4U08LS1FTkQgUFVCTElDIEtFWS0tLS0t", value = "Public key to encrypt data.")
public String getPublicKeyBase64() {
return publicKeyBase64;
}
public void setPublicKeyBase64(String publicKeyBase64) {
this.publicKeyBase64 = publicKeyBase64;
}
public AccessTokenResponse algorithm(String algorithm) {
this.algorithm = algorithm;
return this;
}
/**
* Encyption algorithym. One way ECDH 256 bit key.
* @return algorithm
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "RSA/NONE/PKCS1PADDING", value = "Encyption algorithym. One way ECDH 256 bit key.")
public String getAlgorithm() {
return algorithm;
}
public void setAlgorithm(String algorithm) {
this.algorithm = algorithm;
}
public AccessTokenResponse clientRequestId(String clientRequestId) {
this.clientRequestId = clientRequestId;
return this;
}
/**
* Echoes back the value from the request header for tracking.
* @return clientRequestId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "30dd879c-ee2f-11db-8314-0800200c9a66", value = "Echoes back the value from the request header for tracking.")
public String getClientRequestId() {
return clientRequestId;
}
public void setClientRequestId(String clientRequestId) {
this.clientRequestId = clientRequestId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AccessTokenResponse accessTokenResponse = (AccessTokenResponse) o;
return Objects.equals(this.tokenId, accessTokenResponse.tokenId) &&
Objects.equals(this.status, accessTokenResponse.status) &&
Objects.equals(this.issuedOn, accessTokenResponse.issuedOn) &&
Objects.equals(this.expiresInSeconds, accessTokenResponse.expiresInSeconds) &&
Objects.equals(this.publicKeyBase64, accessTokenResponse.publicKeyBase64) &&
Objects.equals(this.algorithm, accessTokenResponse.algorithm) &&
Objects.equals(this.clientRequestId, accessTokenResponse.clientRequestId);
}
@Override
public int hashCode() {
return Objects.hash(tokenId, status, issuedOn, expiresInSeconds, publicKeyBase64, algorithm, clientRequestId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AccessTokenResponse {\n");
sb.append(" tokenId: ").append(toIndentedString(tokenId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" issuedOn: ").append(toIndentedString(issuedOn)).append("\n");
sb.append(" expiresInSeconds: ").append(toIndentedString(expiresInSeconds)).append("\n");
sb.append(" publicKeyBase64: ").append(toIndentedString(publicKeyBase64)).append("\n");
sb.append(" algorithm: ").append(toIndentedString(algorithm)).append("\n");
sb.append(" clientRequestId: ").append(toIndentedString(clientRequestId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy