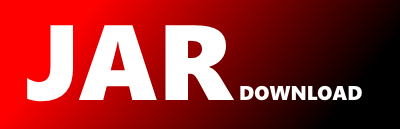
com.github.GBSEcom.model.AdditionalDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.github.GBSEcom.model.ReceiptRequestInfo;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Merchant supplied tracking numbers.
*/
@ApiModel(description = "Merchant supplied tracking numbers.")
public class AdditionalDetails {
public static final String SERIALIZED_NAME_COMMENTS = "comments";
@SerializedName(SERIALIZED_NAME_COMMENTS)
private String comments;
public static final String SERIALIZED_NAME_INVOICE_NUMBER = "invoiceNumber";
@SerializedName(SERIALIZED_NAME_INVOICE_NUMBER)
private String invoiceNumber;
public static final String SERIALIZED_NAME_PURCHASE_ORDER_NUMBER = "purchaseOrderNumber";
@SerializedName(SERIALIZED_NAME_PURCHASE_ORDER_NUMBER)
private String purchaseOrderNumber;
public static final String SERIALIZED_NAME_OPERATOR_ID = "operatorId";
@SerializedName(SERIALIZED_NAME_OPERATOR_ID)
private String operatorId;
public static final String SERIALIZED_NAME_SALES_SYSTEM_ID = "salesSystemId";
@SerializedName(SERIALIZED_NAME_SALES_SYSTEM_ID)
private String salesSystemId;
public static final String SERIALIZED_NAME_IPG_DEFERRED_AUTH = "ipgDeferredAuth";
@SerializedName(SERIALIZED_NAME_IPG_DEFERRED_AUTH)
private Boolean ipgDeferredAuth;
public static final String SERIALIZED_NAME_HIGH_RISK_PURCHASE_INDICATOR = "highRiskPurchaseIndicator";
@SerializedName(SERIALIZED_NAME_HIGH_RISK_PURCHASE_INDICATOR)
private Boolean highRiskPurchaseIndicator;
public static final String SERIALIZED_NAME_RECEIPTS = "receipts";
@SerializedName(SERIALIZED_NAME_RECEIPTS)
private List receipts = null;
/**
* Strong customer authentication exemption type indicator.
*/
@JsonAdapter(ScaExemptionTypeEnum.Adapter.class)
public enum ScaExemptionTypeEnum {
LOW_VALUE_EXEMPTION("Low Value Exemption"),
TRA_EXEMPTION("TRA Exemption"),
TRUSTED_MERCHANT_EXEMPTION("Trusted Merchant Exemption"),
SCP_EXEMPTION("SCP Exemption"),
DELEGATED_AUTHENTICATION("Delegated Authentication");
private String value;
ScaExemptionTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ScaExemptionTypeEnum fromValue(String value) {
for (ScaExemptionTypeEnum b : ScaExemptionTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ScaExemptionTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ScaExemptionTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ScaExemptionTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_SCA_EXEMPTION_TYPE = "scaExemptionType";
@SerializedName(SERIALIZED_NAME_SCA_EXEMPTION_TYPE)
private ScaExemptionTypeEnum scaExemptionType;
public static final String SERIALIZED_NAME_SCA_VISA_MERCHANT_I_D = "scaVisaMerchantID";
@SerializedName(SERIALIZED_NAME_SCA_VISA_MERCHANT_I_D)
private String scaVisaMerchantID;
public AdditionalDetails comments(String comments) {
this.comments = comments;
return this;
}
/**
* Comments for the payment.
* @return comments
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "This is a comment", value = "Comments for the payment.")
public String getComments() {
return comments;
}
public void setComments(String comments) {
this.comments = comments;
}
public AdditionalDetails invoiceNumber(String invoiceNumber) {
this.invoiceNumber = invoiceNumber;
return this;
}
/**
* Invoice number.
* @return invoiceNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "551294633441", value = "Invoice number.")
public String getInvoiceNumber() {
return invoiceNumber;
}
public void setInvoiceNumber(String invoiceNumber) {
this.invoiceNumber = invoiceNumber;
}
public AdditionalDetails purchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
return this;
}
/**
* Purchase order number.
* @return purchaseOrderNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1223456", value = "Purchase order number.")
public String getPurchaseOrderNumber() {
return purchaseOrderNumber;
}
public void setPurchaseOrderNumber(String purchaseOrderNumber) {
this.purchaseOrderNumber = purchaseOrderNumber;
}
public AdditionalDetails operatorId(String operatorId) {
this.operatorId = operatorId;
return this;
}
/**
* The operator ID.
* @return operatorId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The operator ID.")
public String getOperatorId() {
return operatorId;
}
public void setOperatorId(String operatorId) {
this.operatorId = operatorId;
}
public AdditionalDetails salesSystemId(String salesSystemId) {
this.salesSystemId = salesSystemId;
return this;
}
/**
* The sales system ID.
* @return salesSystemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The sales system ID.")
public String getSalesSystemId() {
return salesSystemId;
}
public void setSalesSystemId(String salesSystemId) {
this.salesSystemId = salesSystemId;
}
public AdditionalDetails ipgDeferredAuth(Boolean ipgDeferredAuth) {
this.ipgDeferredAuth = ipgDeferredAuth;
return this;
}
/**
* Indicates if the particular transaction is a deferred authorization.
* @return ipgDeferredAuth
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "true", value = "Indicates if the particular transaction is a deferred authorization.")
public Boolean getIpgDeferredAuth() {
return ipgDeferredAuth;
}
public void setIpgDeferredAuth(Boolean ipgDeferredAuth) {
this.ipgDeferredAuth = ipgDeferredAuth;
}
public AdditionalDetails highRiskPurchaseIndicator(Boolean highRiskPurchaseIndicator) {
this.highRiskPurchaseIndicator = highRiskPurchaseIndicator;
return this;
}
/**
* this is highRiskPurchaseIndicator.
* @return highRiskPurchaseIndicator
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "true", value = "this is highRiskPurchaseIndicator.")
public Boolean getHighRiskPurchaseIndicator() {
return highRiskPurchaseIndicator;
}
public void setHighRiskPurchaseIndicator(Boolean highRiskPurchaseIndicator) {
this.highRiskPurchaseIndicator = highRiskPurchaseIndicator;
}
public AdditionalDetails receipts(List receipts) {
this.receipts = receipts;
return this;
}
public AdditionalDetails addReceiptsItem(ReceiptRequestInfo receiptsItem) {
if (this.receipts == null) {
this.receipts = new ArrayList<>();
}
this.receipts.add(receiptsItem);
return this;
}
/**
* Provides request information that is necessary to generate receipts.
* @return receipts
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[{\"type\":\"cardholder\",\"locale\":\"de-DE\"},{\"type\":\"merchant\",\"locale\":\"en\",\"linewidth\":48}]", value = "Provides request information that is necessary to generate receipts.")
public List getReceipts() {
return receipts;
}
public void setReceipts(List receipts) {
this.receipts = receipts;
}
public AdditionalDetails scaExemptionType(ScaExemptionTypeEnum scaExemptionType) {
this.scaExemptionType = scaExemptionType;
return this;
}
/**
* Strong customer authentication exemption type indicator.
* @return scaExemptionType
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "TRA Exemption", value = "Strong customer authentication exemption type indicator.")
public ScaExemptionTypeEnum getScaExemptionType() {
return scaExemptionType;
}
public void setScaExemptionType(ScaExemptionTypeEnum scaExemptionType) {
this.scaExemptionType = scaExemptionType;
}
public AdditionalDetails scaVisaMerchantID(String scaVisaMerchantID) {
this.scaVisaMerchantID = scaVisaMerchantID;
return this;
}
/**
* Eight-character Visa merchant identifier (VMID) assigned by Visa, required for trusted merchant and delegated authentication.
* @return scaVisaMerchantID
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "12312311", value = "Eight-character Visa merchant identifier (VMID) assigned by Visa, required for trusted merchant and delegated authentication.")
public String getScaVisaMerchantID() {
return scaVisaMerchantID;
}
public void setScaVisaMerchantID(String scaVisaMerchantID) {
this.scaVisaMerchantID = scaVisaMerchantID;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AdditionalDetails additionalDetails = (AdditionalDetails) o;
return Objects.equals(this.comments, additionalDetails.comments) &&
Objects.equals(this.invoiceNumber, additionalDetails.invoiceNumber) &&
Objects.equals(this.purchaseOrderNumber, additionalDetails.purchaseOrderNumber) &&
Objects.equals(this.operatorId, additionalDetails.operatorId) &&
Objects.equals(this.salesSystemId, additionalDetails.salesSystemId) &&
Objects.equals(this.ipgDeferredAuth, additionalDetails.ipgDeferredAuth) &&
Objects.equals(this.highRiskPurchaseIndicator, additionalDetails.highRiskPurchaseIndicator) &&
Objects.equals(this.receipts, additionalDetails.receipts) &&
Objects.equals(this.scaExemptionType, additionalDetails.scaExemptionType) &&
Objects.equals(this.scaVisaMerchantID, additionalDetails.scaVisaMerchantID);
}
@Override
public int hashCode() {
return Objects.hash(comments, invoiceNumber, purchaseOrderNumber, operatorId, salesSystemId, ipgDeferredAuth, highRiskPurchaseIndicator, receipts, scaExemptionType, scaVisaMerchantID);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AdditionalDetails {\n");
sb.append(" comments: ").append(toIndentedString(comments)).append("\n");
sb.append(" invoiceNumber: ").append(toIndentedString(invoiceNumber)).append("\n");
sb.append(" purchaseOrderNumber: ").append(toIndentedString(purchaseOrderNumber)).append("\n");
sb.append(" operatorId: ").append(toIndentedString(operatorId)).append("\n");
sb.append(" salesSystemId: ").append(toIndentedString(salesSystemId)).append("\n");
sb.append(" ipgDeferredAuth: ").append(toIndentedString(ipgDeferredAuth)).append("\n");
sb.append(" highRiskPurchaseIndicator: ").append(toIndentedString(highRiskPurchaseIndicator)).append("\n");
sb.append(" receipts: ").append(toIndentedString(receipts)).append("\n");
sb.append(" scaExemptionType: ").append(toIndentedString(scaExemptionType)).append("\n");
sb.append(" scaVisaMerchantID: ").append(toIndentedString(scaVisaMerchantID)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy