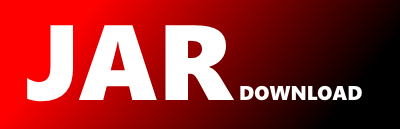
com.github.GBSEcom.model.FraudOrder Maven / Gradle / Ivy
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.github.GBSEcom.model.FraudOrderItems;
import com.github.GBSEcom.model.ShipToAddress;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* The list of items included in the order.
*/
@ApiModel(description = "The list of items included in the order.")
public class FraudOrder {
public static final String SERIALIZED_NAME_SHIP_TO_ADDRESS = "shipToAddress";
@SerializedName(SERIALIZED_NAME_SHIP_TO_ADDRESS)
private ShipToAddress shipToAddress;
public static final String SERIALIZED_NAME_ITEMS = "items";
@SerializedName(SERIALIZED_NAME_ITEMS)
private List items = null;
public static final String SERIALIZED_NAME_DETAILS_URL = "detailsUrl";
@SerializedName(SERIALIZED_NAME_DETAILS_URL)
private String detailsUrl;
public static final String SERIALIZED_NAME_USER_DEFINED = "userDefined";
@SerializedName(SERIALIZED_NAME_USER_DEFINED)
private Object userDefined;
public FraudOrder shipToAddress(ShipToAddress shipToAddress) {
this.shipToAddress = shipToAddress;
return this;
}
/**
* Get shipToAddress
* @return shipToAddress
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ShipToAddress getShipToAddress() {
return shipToAddress;
}
public void setShipToAddress(ShipToAddress shipToAddress) {
this.shipToAddress = shipToAddress;
}
public FraudOrder items(List items) {
this.items = items;
return this;
}
public FraudOrder addItemsItem(FraudOrderItems itemsItem) {
if (this.items == null) {
this.items = new ArrayList<>();
}
this.items.add(itemsItem);
return this;
}
/**
* The list of items included in the order.
* @return items
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[{\"id\":\"PRODCODE1\",\"name\":\"The Art of Computer Programming\",\"quantity\":\"litre\",\"unit\":\"1\",\"unitPrice\":{\"value\":7300,\"currency\":\"USD\"},\"categories\":\"[[\\\"Books\\\", \\\"Computers & Technology\\\", \\\"Programming\\\"], [\\\"Books\\\", \\\"Text Books\\\", \\\"Computer Science\\\"]]\",\"detailsUrl\":\"https://mystore.domain/product/PRODCODE1\",\"userDefined\":{\"weight\":5021.23,\"vat\":0.06}}]", value = "The list of items included in the order.")
public List getItems() {
return items;
}
public void setItems(List items) {
this.items = items;
}
public FraudOrder detailsUrl(String detailsUrl) {
this.detailsUrl = detailsUrl;
return this;
}
/**
* The URL to the merchant's management system, for reporting and analysis.
* @return detailsUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "https://mystore.domain/product/PRODCODE1", value = "The URL to the merchant's management system, for reporting and analysis.")
public String getDetailsUrl() {
return detailsUrl;
}
public void setDetailsUrl(String detailsUrl) {
this.detailsUrl = detailsUrl;
}
public FraudOrder userDefined(Object userDefined) {
this.userDefined = userDefined;
return this;
}
/**
* A JSON object that can carry any additional information about the order that might be helpful for fraud detection.
* @return userDefined
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "{\"delivery\":\"express\",\"carrier\":\"ups\"}", value = "A JSON object that can carry any additional information about the order that might be helpful for fraud detection.")
public Object getUserDefined() {
return userDefined;
}
public void setUserDefined(Object userDefined) {
this.userDefined = userDefined;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
FraudOrder fraudOrder = (FraudOrder) o;
return Objects.equals(this.shipToAddress, fraudOrder.shipToAddress) &&
Objects.equals(this.items, fraudOrder.items) &&
Objects.equals(this.detailsUrl, fraudOrder.detailsUrl) &&
Objects.equals(this.userDefined, fraudOrder.userDefined);
}
@Override
public int hashCode() {
return Objects.hash(shipToAddress, items, detailsUrl, userDefined);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class FraudOrder {\n");
sb.append(" shipToAddress: ").append(toIndentedString(shipToAddress)).append("\n");
sb.append(" items: ").append(toIndentedString(items)).append("\n");
sb.append(" detailsUrl: ").append(toIndentedString(detailsUrl)).append("\n");
sb.append(" userDefined: ").append(toIndentedString(userDefined)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy