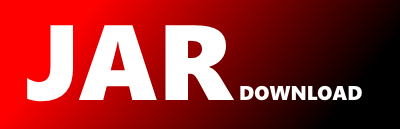
com.github.GBSEcom.model.IssuerResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* The issuers response to the payment request. This field should be filled in when the message has already passed through the issuer (e.g. post-authorization).
*/
@ApiModel(description = "The issuers response to the payment request. This field should be filled in when the message has already passed through the issuer (e.g. post-authorization).")
public class IssuerResponse {
public static final String SERIALIZED_NAME_CODE = "code";
@SerializedName(SERIALIZED_NAME_CODE)
private String code;
/**
* The interpretation of the response code. Valid values are \"approved\" - The verification was conducted and is approved. \"declined\" - The verification was conducted and is not approved. \"disabled\" - The verification was not conducted because it was not requested or disabled in the verification. \"unknown\" - The verification was attempted but it failed due to some system error (e.g. timeout).
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
APPROVED("approved"),
DECLINED("declined"),
DISABLED("disabled"),
UNKNOWN("unknown");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String value) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private StatusEnum status;
public static final String SERIALIZED_NAME_SCHEME = "scheme";
@SerializedName(SERIALIZED_NAME_SCHEME)
private String scheme;
public IssuerResponse code(String code) {
this.code = code;
return this;
}
/**
* The verification response code, as sent by the verification system.
* @return code
**/
@ApiModelProperty(example = "100", required = true, value = "The verification response code, as sent by the verification system.")
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public IssuerResponse status(StatusEnum status) {
this.status = status;
return this;
}
/**
* The interpretation of the response code. Valid values are \"approved\" - The verification was conducted and is approved. \"declined\" - The verification was conducted and is not approved. \"disabled\" - The verification was not conducted because it was not requested or disabled in the verification. \"unknown\" - The verification was attempted but it failed due to some system error (e.g. timeout).
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "approved", value = "The interpretation of the response code. Valid values are \"approved\" - The verification was conducted and is approved. \"declined\" - The verification was conducted and is not approved. \"disabled\" - The verification was not conducted because it was not requested or disabled in the verification. \"unknown\" - The verification was attempted but it failed due to some system error (e.g. timeout).")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public IssuerResponse scheme(String scheme) {
this.scheme = scheme;
return this;
}
/**
* An identifier of the system/specification from which the code was received, and how the status was derived.
* @return scheme
**/
@ApiModelProperty(example = "visa", required = true, value = "An identifier of the system/specification from which the code was received, and how the status was derived.")
public String getScheme() {
return scheme;
}
public void setScheme(String scheme) {
this.scheme = scheme;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
IssuerResponse issuerResponse = (IssuerResponse) o;
return Objects.equals(this.code, issuerResponse.code) &&
Objects.equals(this.status, issuerResponse.status) &&
Objects.equals(this.scheme, issuerResponse.scheme);
}
@Override
public int hashCode() {
return Objects.hash(code, status, scheme);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class IssuerResponse {\n");
sb.append(" code: ").append(toIndentedString(code)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" scheme: ").append(toIndentedString(scheme)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy