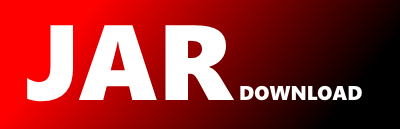
com.github.GBSEcom.model.PaymentDevice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.github.GBSEcom.model.CardFunction;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Information from the payment device including the blob data and its mode of entry.
*/
@ApiModel(description = "Information from the payment device including the blob data and its mode of entry.")
public class PaymentDevice {
/**
* The data format.
*/
@JsonAdapter(DeviceTypeEnum.Adapter.class)
public enum DeviceTypeEnum {
SWIPE("SWIPE");
private String value;
DeviceTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DeviceTypeEnum fromValue(String value) {
for (DeviceTypeEnum b : DeviceTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DeviceTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DeviceTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DeviceTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DEVICE_TYPE = "deviceType";
@SerializedName(SERIALIZED_NAME_DEVICE_TYPE)
private DeviceTypeEnum deviceType;
public static final String SERIALIZED_NAME_DATA = "data";
@SerializedName(SERIALIZED_NAME_DATA)
private String data;
public static final String SERIALIZED_NAME_SECURITY_CODE = "securityCode";
@SerializedName(SERIALIZED_NAME_SECURITY_CODE)
private String securityCode;
public static final String SERIALIZED_NAME_CARDHOLDER_NAME = "cardholderName";
@SerializedName(SERIALIZED_NAME_CARDHOLDER_NAME)
private String cardholderName;
public static final String SERIALIZED_NAME_CARD_FUNCTION = "cardFunction";
@SerializedName(SERIALIZED_NAME_CARD_FUNCTION)
private CardFunction cardFunction;
public static final String SERIALIZED_NAME_BRAND = "brand";
@SerializedName(SERIALIZED_NAME_BRAND)
private String brand;
public PaymentDevice deviceType(DeviceTypeEnum deviceType) {
this.deviceType = deviceType;
return this;
}
/**
* The data format.
* @return deviceType
**/
@ApiModelProperty(example = "SWIPE", required = true, value = "The data format.")
public DeviceTypeEnum getDeviceType() {
return deviceType;
}
public void setDeviceType(DeviceTypeEnum deviceType) {
this.deviceType = deviceType;
}
public PaymentDevice data(String data) {
this.data = data;
return this;
}
/**
* Data from device containing, at a minimum, a transaction-unique key serial number (KSN) and track 2 card data.
* @return data
**/
@ApiModelProperty(example = "02A600C0170018008292;5424********1732=1810?*B73CD8C26233D4FFEC5500ED394439D97DDA5F530942D21D0000000000000000000000000000000000000000363434543035353734326299492410027300000260DC03", required = true, value = "Data from device containing, at a minimum, a transaction-unique key serial number (KSN) and track 2 card data.")
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
public PaymentDevice securityCode(String securityCode) {
this.securityCode = securityCode;
return this;
}
/**
* Card verification value/number.
* @return securityCode
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "977", value = "Card verification value/number.")
public String getSecurityCode() {
return securityCode;
}
public void setSecurityCode(String securityCode) {
this.securityCode = securityCode;
}
public PaymentDevice cardholderName(String cardholderName) {
this.cardholderName = cardholderName;
return this;
}
/**
* Name of cardholder.
* @return cardholderName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of cardholder.")
public String getCardholderName() {
return cardholderName;
}
public void setCardholderName(String cardholderName) {
this.cardholderName = cardholderName;
}
public PaymentDevice cardFunction(CardFunction cardFunction) {
this.cardFunction = cardFunction;
return this;
}
/**
* Get cardFunction
* @return cardFunction
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public CardFunction getCardFunction() {
return cardFunction;
}
public void setCardFunction(CardFunction cardFunction) {
this.cardFunction = cardFunction;
}
public PaymentDevice brand(String brand) {
this.brand = brand;
return this;
}
/**
* The card brand.
* @return brand
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "VISA", value = "The card brand.")
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentDevice paymentDevice = (PaymentDevice) o;
return Objects.equals(this.deviceType, paymentDevice.deviceType) &&
Objects.equals(this.data, paymentDevice.data) &&
Objects.equals(this.securityCode, paymentDevice.securityCode) &&
Objects.equals(this.cardholderName, paymentDevice.cardholderName) &&
Objects.equals(this.cardFunction, paymentDevice.cardFunction) &&
Objects.equals(this.brand, paymentDevice.brand);
}
@Override
public int hashCode() {
return Objects.hash(deviceType, data, securityCode, cardholderName, cardFunction, brand);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentDevice {\n");
sb.append(" deviceType: ").append(toIndentedString(deviceType)).append("\n");
sb.append(" data: ").append(toIndentedString(data)).append("\n");
sb.append(" securityCode: ").append(toIndentedString(securityCode)).append("\n");
sb.append(" cardholderName: ").append(toIndentedString(cardholderName)).append("\n");
sb.append(" cardFunction: ").append(toIndentedString(cardFunction)).append("\n");
sb.append(" brand: ").append(toIndentedString(brand)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy