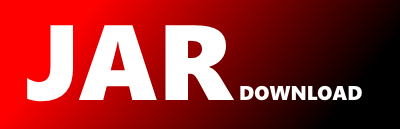
com.github.GBSEcom.model.ProvideDetailPaymentStepRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.github.GBSEcom.model.PaymentStepRequest;
import com.github.GBSEcom.model.ProvideDetailPaymentStepRequestAllOf;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Request to inquire information from the payer or merchant.
*/
@ApiModel(description = "Request to inquire information from the payer or merchant.")
public class ProvideDetailPaymentStepRequest {
public static final String SERIALIZED_NAME_TYPE = "type";
@SerializedName(SERIALIZED_NAME_TYPE)
private String type;
public static final String SERIALIZED_NAME_HINT = "hint";
@SerializedName(SERIALIZED_NAME_HINT)
private String hint;
public static final String SERIALIZED_NAME_JAVA_SCRIPT_VALIDATION_EXPRESSION = "javaScriptValidationExpression";
@SerializedName(SERIALIZED_NAME_JAVA_SCRIPT_VALIDATION_EXPRESSION)
private String javaScriptValidationExpression;
public static final String SERIALIZED_NAME_KEY = "key";
@SerializedName(SERIALIZED_NAME_KEY)
private String key;
public static final String SERIALIZED_NAME_LABEL = "label";
@SerializedName(SERIALIZED_NAME_LABEL)
private String label;
public ProvideDetailPaymentStepRequest type(String type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@ApiModelProperty(example = "provide-detail", required = true, value = "")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public ProvideDetailPaymentStepRequest hint(String hint) {
this.hint = hint;
return this;
}
/**
* Get hint
* @return hint
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getHint() {
return hint;
}
public void setHint(String hint) {
this.hint = hint;
}
public ProvideDetailPaymentStepRequest javaScriptValidationExpression(String javaScriptValidationExpression) {
this.javaScriptValidationExpression = javaScriptValidationExpression;
return this;
}
/**
* Get javaScriptValidationExpression
* @return javaScriptValidationExpression
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getJavaScriptValidationExpression() {
return javaScriptValidationExpression;
}
public void setJavaScriptValidationExpression(String javaScriptValidationExpression) {
this.javaScriptValidationExpression = javaScriptValidationExpression;
}
public ProvideDetailPaymentStepRequest key(String key) {
this.key = key;
return this;
}
/**
* Get key
* @return key
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getKey() {
return key;
}
public void setKey(String key) {
this.key = key;
}
public ProvideDetailPaymentStepRequest label(String label) {
this.label = label;
return this;
}
/**
* Get label
* @return label
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ProvideDetailPaymentStepRequest provideDetailPaymentStepRequest = (ProvideDetailPaymentStepRequest) o;
return Objects.equals(this.type, provideDetailPaymentStepRequest.type) &&
Objects.equals(this.hint, provideDetailPaymentStepRequest.hint) &&
Objects.equals(this.javaScriptValidationExpression, provideDetailPaymentStepRequest.javaScriptValidationExpression) &&
Objects.equals(this.key, provideDetailPaymentStepRequest.key) &&
Objects.equals(this.label, provideDetailPaymentStepRequest.label);
}
@Override
public int hashCode() {
return Objects.hash(type, hint, javaScriptValidationExpression, key, label);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ProvideDetailPaymentStepRequest {\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" hint: ").append(toIndentedString(hint)).append("\n");
sb.append(" javaScriptValidationExpression: ").append(toIndentedString(javaScriptValidationExpression)).append("\n");
sb.append(" key: ").append(toIndentedString(key)).append("\n");
sb.append(" label: ").append(toIndentedString(label)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy