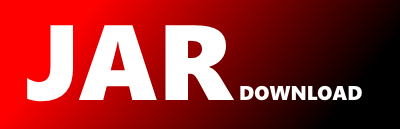
com.github.GBSEcom.model.PurchaseCards Maven / Gradle / Ivy
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.github.GBSEcom.model.PurchaseCardsLevel2;
import com.github.GBSEcom.model.PurchaseCardsLevel3;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Purchase card details.
*/
@ApiModel(description = "Purchase card details.")
public class PurchaseCards {
public static final String SERIALIZED_NAME_LEVEL2 = "Level2";
@SerializedName(SERIALIZED_NAME_LEVEL2)
private PurchaseCardsLevel2 level2;
public static final String SERIALIZED_NAME_LEVEL3 = "Level3";
@SerializedName(SERIALIZED_NAME_LEVEL3)
private PurchaseCardsLevel3 level3;
public PurchaseCards level2(PurchaseCardsLevel2 level2) {
this.level2 = level2;
return this;
}
/**
* Get level2
* @return level2
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PurchaseCardsLevel2 getLevel2() {
return level2;
}
public void setLevel2(PurchaseCardsLevel2 level2) {
this.level2 = level2;
}
public PurchaseCards level3(PurchaseCardsLevel3 level3) {
this.level3 = level3;
return this;
}
/**
* Get level3
* @return level3
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PurchaseCardsLevel3 getLevel3() {
return level3;
}
public void setLevel3(PurchaseCardsLevel3 level3) {
this.level3 = level3;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PurchaseCards purchaseCards = (PurchaseCards) o;
return Objects.equals(this.level2, purchaseCards.level2) &&
Objects.equals(this.level3, purchaseCards.level3);
}
@Override
public int hashCode() {
return Objects.hash(level2, level3);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PurchaseCards {\n");
sb.append(" level2: ").append(toIndentedString(level2)).append("\n");
sb.append(" level3: ").append(toIndentedString(level3)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy