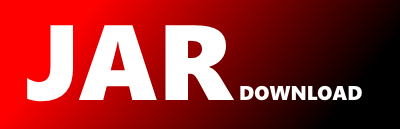
com.github.GBSEcom.model.PurchaseCardsLevel2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.github.GBSEcom.model.AdditionalAmountRate;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Level 2 data for monitoring and controlling corporate expenditures.
*/
@ApiModel(description = "Level 2 data for monitoring and controlling corporate expenditures.")
public class PurchaseCardsLevel2 {
public static final String SERIALIZED_NAME_CUSTOMER_REFERENCE_I_D = "customerReferenceID";
@SerializedName(SERIALIZED_NAME_CUSTOMER_REFERENCE_I_D)
private String customerReferenceID;
public static final String SERIALIZED_NAME_SUPPLIER_INVOICE_NUMBER = "supplierInvoiceNumber";
@SerializedName(SERIALIZED_NAME_SUPPLIER_INVOICE_NUMBER)
private String supplierInvoiceNumber;
public static final String SERIALIZED_NAME_SUPPLIER_V_A_T_REGISTRATION_NUMBER = "supplierVATRegistrationNumber";
@SerializedName(SERIALIZED_NAME_SUPPLIER_V_A_T_REGISTRATION_NUMBER)
private String supplierVATRegistrationNumber;
public static final String SERIALIZED_NAME_TOTAL_DISCOUNT_AMOUNT_AND_RATE = "totalDiscountAmountAndRate";
@SerializedName(SERIALIZED_NAME_TOTAL_DISCOUNT_AMOUNT_AND_RATE)
private AdditionalAmountRate totalDiscountAmountAndRate;
public static final String SERIALIZED_NAME_VAT_SHIPPING_AMOUNT_AND_RATE = "vatShippingAmountAndRate";
@SerializedName(SERIALIZED_NAME_VAT_SHIPPING_AMOUNT_AND_RATE)
private AdditionalAmountRate vatShippingAmountAndRate;
public PurchaseCardsLevel2 customerReferenceID(String customerReferenceID) {
this.customerReferenceID = customerReferenceID;
return this;
}
/**
* Customer code/customer reference ID. The max length supported for Visa is 12 and MasterCard is 17.
* @return customerReferenceID
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "abcdef123xyz", value = "Customer code/customer reference ID. The max length supported for Visa is 12 and MasterCard is 17.")
public String getCustomerReferenceID() {
return customerReferenceID;
}
public void setCustomerReferenceID(String customerReferenceID) {
this.customerReferenceID = customerReferenceID;
}
public PurchaseCardsLevel2 supplierInvoiceNumber(String supplierInvoiceNumber) {
this.supplierInvoiceNumber = supplierInvoiceNumber;
return this;
}
/**
* Purchase identifier/merchant-related data.
* @return supplierInvoiceNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "0000000065348", value = "Purchase identifier/merchant-related data.")
public String getSupplierInvoiceNumber() {
return supplierInvoiceNumber;
}
public void setSupplierInvoiceNumber(String supplierInvoiceNumber) {
this.supplierInvoiceNumber = supplierInvoiceNumber;
}
public PurchaseCardsLevel2 supplierVATRegistrationNumber(String supplierVATRegistrationNumber) {
this.supplierVATRegistrationNumber = supplierVATRegistrationNumber;
return this;
}
/**
* Merchant VAT registration/single business reference number/merchant tax ID or corporation VAT number.
* @return supplierVATRegistrationNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "10001174242", value = "Merchant VAT registration/single business reference number/merchant tax ID or corporation VAT number.")
public String getSupplierVATRegistrationNumber() {
return supplierVATRegistrationNumber;
}
public void setSupplierVATRegistrationNumber(String supplierVATRegistrationNumber) {
this.supplierVATRegistrationNumber = supplierVATRegistrationNumber;
}
public PurchaseCardsLevel2 totalDiscountAmountAndRate(AdditionalAmountRate totalDiscountAmountAndRate) {
this.totalDiscountAmountAndRate = totalDiscountAmountAndRate;
return this;
}
/**
* Get totalDiscountAmountAndRate
* @return totalDiscountAmountAndRate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public AdditionalAmountRate getTotalDiscountAmountAndRate() {
return totalDiscountAmountAndRate;
}
public void setTotalDiscountAmountAndRate(AdditionalAmountRate totalDiscountAmountAndRate) {
this.totalDiscountAmountAndRate = totalDiscountAmountAndRate;
}
public PurchaseCardsLevel2 vatShippingAmountAndRate(AdditionalAmountRate vatShippingAmountAndRate) {
this.vatShippingAmountAndRate = vatShippingAmountAndRate;
return this;
}
/**
* Get vatShippingAmountAndRate
* @return vatShippingAmountAndRate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public AdditionalAmountRate getVatShippingAmountAndRate() {
return vatShippingAmountAndRate;
}
public void setVatShippingAmountAndRate(AdditionalAmountRate vatShippingAmountAndRate) {
this.vatShippingAmountAndRate = vatShippingAmountAndRate;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PurchaseCardsLevel2 purchaseCardsLevel2 = (PurchaseCardsLevel2) o;
return Objects.equals(this.customerReferenceID, purchaseCardsLevel2.customerReferenceID) &&
Objects.equals(this.supplierInvoiceNumber, purchaseCardsLevel2.supplierInvoiceNumber) &&
Objects.equals(this.supplierVATRegistrationNumber, purchaseCardsLevel2.supplierVATRegistrationNumber) &&
Objects.equals(this.totalDiscountAmountAndRate, purchaseCardsLevel2.totalDiscountAmountAndRate) &&
Objects.equals(this.vatShippingAmountAndRate, purchaseCardsLevel2.vatShippingAmountAndRate);
}
@Override
public int hashCode() {
return Objects.hash(customerReferenceID, supplierInvoiceNumber, supplierVATRegistrationNumber, totalDiscountAmountAndRate, vatShippingAmountAndRate);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PurchaseCardsLevel2 {\n");
sb.append(" customerReferenceID: ").append(toIndentedString(customerReferenceID)).append("\n");
sb.append(" supplierInvoiceNumber: ").append(toIndentedString(supplierInvoiceNumber)).append("\n");
sb.append(" supplierVATRegistrationNumber: ").append(toIndentedString(supplierVATRegistrationNumber)).append("\n");
sb.append(" totalDiscountAmountAndRate: ").append(toIndentedString(totalDiscountAmountAndRate)).append("\n");
sb.append(" vatShippingAmountAndRate: ").append(toIndentedString(vatShippingAmountAndRate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy