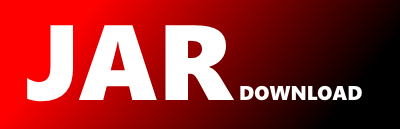
com.github.GBSEcom.model.ScoreOnlyResponseFraudScore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.github.GBSEcom.model.ScoreOnlyResponseFraudScoreExplanations;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Fraud likelihood assessment consisting of a score, associated warning(s), and explanation(s) of score received.
*/
@ApiModel(description = "Fraud likelihood assessment consisting of a score, associated warning(s), and explanation(s) of score received.")
public class ScoreOnlyResponseFraudScore {
public static final String SERIALIZED_NAME_SCORE = "score";
@SerializedName(SERIALIZED_NAME_SCORE)
private String score;
public static final String SERIALIZED_NAME_WARNINGS = "warnings";
@SerializedName(SERIALIZED_NAME_WARNINGS)
private List warnings = null;
public static final String SERIALIZED_NAME_EXPLANATIONS = "explanations";
@SerializedName(SERIALIZED_NAME_EXPLANATIONS)
private List explanations = null;
public static final String SERIALIZED_NAME_RECOMMENDED_DECISION = "recommendedDecision";
@SerializedName(SERIALIZED_NAME_RECOMMENDED_DECISION)
private String recommendedDecision;
public ScoreOnlyResponseFraudScore score(String score) {
this.score = score;
return this;
}
/**
* The score attributed to this request by our machine learning system, ranging from 0 (less likely to be fraud) to 1000 (more likely to be fraud).
* @return score
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1000", value = "The score attributed to this request by our machine learning system, ranging from 0 (less likely to be fraud) to 1000 (more likely to be fraud).")
public String getScore() {
return score;
}
public void setScore(String score) {
this.score = score;
}
public ScoreOnlyResponseFraudScore warnings(List warnings) {
this.warnings = warnings;
return this;
}
public ScoreOnlyResponseFraudScore addWarningsItem(String warningsItem) {
if (this.warnings == null) {
this.warnings = new ArrayList<>();
}
this.warnings.add(warningsItem);
return this;
}
/**
* A list of non-critical warnings raised while processing the request. Warnings included in this list will have integration and data-quality related messages.
* @return warnings
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"warning1\",\"warning2\"]", value = "A list of non-critical warnings raised while processing the request. Warnings included in this list will have integration and data-quality related messages.")
public List getWarnings() {
return warnings;
}
public void setWarnings(List warnings) {
this.warnings = warnings;
}
public ScoreOnlyResponseFraudScore explanations(List explanations) {
this.explanations = explanations;
return this;
}
public ScoreOnlyResponseFraudScore addExplanationsItem(ScoreOnlyResponseFraudScoreExplanations explanationsItem) {
if (this.explanations == null) {
this.explanations = new ArrayList<>();
}
this.explanations.add(explanationsItem);
return this;
}
/**
* Explanation of the fraud score applied consisting of a description, type of the explanation, and rule (if applicable).
* @return explanations
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[{\"description\":\"Suspicious transaction amount.\",\"type\":\"explanation/model\"},{\"description\":\"Suspicious pattern compared to number of transactions in the past 1 month for the card.\",\"type\":\"explanation/rule\",\"rule\":\"QSR_14\"}]", value = "Explanation of the fraud score applied consisting of a description, type of the explanation, and rule (if applicable).")
public List getExplanations() {
return explanations;
}
public void setExplanations(List explanations) {
this.explanations = explanations;
}
public ScoreOnlyResponseFraudScore recommendedDecision(String recommendedDecision) {
this.recommendedDecision = recommendedDecision;
return this;
}
/**
* The action that should be taken for the request that was sent.
* @return recommendedDecision
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "accept", value = "The action that should be taken for the request that was sent.")
public String getRecommendedDecision() {
return recommendedDecision;
}
public void setRecommendedDecision(String recommendedDecision) {
this.recommendedDecision = recommendedDecision;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ScoreOnlyResponseFraudScore scoreOnlyResponseFraudScore = (ScoreOnlyResponseFraudScore) o;
return Objects.equals(this.score, scoreOnlyResponseFraudScore.score) &&
Objects.equals(this.warnings, scoreOnlyResponseFraudScore.warnings) &&
Objects.equals(this.explanations, scoreOnlyResponseFraudScore.explanations) &&
Objects.equals(this.recommendedDecision, scoreOnlyResponseFraudScore.recommendedDecision);
}
@Override
public int hashCode() {
return Objects.hash(score, warnings, explanations, recommendedDecision);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ScoreOnlyResponseFraudScore {\n");
sb.append(" score: ").append(toIndentedString(score)).append("\n");
sb.append(" warnings: ").append(toIndentedString(warnings)).append("\n");
sb.append(" explanations: ").append(toIndentedString(explanations)).append("\n");
sb.append(" recommendedDecision: ").append(toIndentedString(recommendedDecision)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy