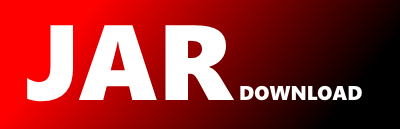
com.github.GBSEcom.model.TeleCheckAchPaymentMethod Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.github.GBSEcom.model.IdInfo;
import com.github.GBSEcom.model.TeleCheckAchPaymentMethodAchBillTo;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.LocalDate;
/**
* ACH means automated clearing house. Contains properties common across TeleCheck message types. Abstract class, do not use this class directly, use one of its children.
*/
@ApiModel(description = "ACH means automated clearing house. Contains properties common across TeleCheck message types. Abstract class, do not use this class directly, use one of its children.")
public class TeleCheckAchPaymentMethod {
public static final String SERIALIZED_NAME_ACH_TYPE = "achType";
@SerializedName(SERIALIZED_NAME_ACH_TYPE)
private String achType;
public static final String SERIALIZED_NAME_ROUTING_NUMBER = "routingNumber";
@SerializedName(SERIALIZED_NAME_ROUTING_NUMBER)
private String routingNumber;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "accountNumber";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
/**
* Identifies if the account type is checking or savings.
*/
@JsonAdapter(AccountTypeEnum.Adapter.class)
public enum AccountTypeEnum {
C("C"),
S("S");
private String value;
AccountTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static AccountTypeEnum fromValue(String value) {
for (AccountTypeEnum b : AccountTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final AccountTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public AccountTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return AccountTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_ACCOUNT_TYPE = "accountType";
@SerializedName(SERIALIZED_NAME_ACCOUNT_TYPE)
private AccountTypeEnum accountType;
public static final String SERIALIZED_NAME_CHECK_NUMBER = "checkNumber";
@SerializedName(SERIALIZED_NAME_CHECK_NUMBER)
private String checkNumber;
/**
* Identifies if the check type is personal or company.
*/
@JsonAdapter(CheckTypeEnum.Adapter.class)
public enum CheckTypeEnum {
P("P"),
C("C");
private String value;
CheckTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static CheckTypeEnum fromValue(String value) {
for (CheckTypeEnum b : CheckTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final CheckTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public CheckTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return CheckTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_CHECK_TYPE = "checkType";
@SerializedName(SERIALIZED_NAME_CHECK_TYPE)
private CheckTypeEnum checkType;
public static final String SERIALIZED_NAME_PRODUCT_CODE = "productCode";
@SerializedName(SERIALIZED_NAME_PRODUCT_CODE)
private String productCode;
public static final String SERIALIZED_NAME_MANUAL_ID_INFO = "manualIdInfo";
@SerializedName(SERIALIZED_NAME_MANUAL_ID_INFO)
private IdInfo manualIdInfo;
public static final String SERIALIZED_NAME_SUPPLEMENT_ID_INFO = "supplementIdInfo";
@SerializedName(SERIALIZED_NAME_SUPPLEMENT_ID_INFO)
private IdInfo supplementIdInfo;
public static final String SERIALIZED_NAME_AGENT_ID = "agentId";
@SerializedName(SERIALIZED_NAME_AGENT_ID)
private String agentId;
public static final String SERIALIZED_NAME_TERMINAL_ID = "terminalId";
@SerializedName(SERIALIZED_NAME_TERMINAL_ID)
private String terminalId;
public static final String SERIALIZED_NAME_REGISTRATION_ID = "registrationId";
@SerializedName(SERIALIZED_NAME_REGISTRATION_ID)
private String registrationId;
public static final String SERIALIZED_NAME_REGISTRATION_DATE = "registrationDate";
@SerializedName(SERIALIZED_NAME_REGISTRATION_DATE)
private LocalDate registrationDate;
/**
* Release type is used as a risk variable to gauge risk level when the merchant is releasing the purchased merchandise.
*/
@JsonAdapter(ReleaseTypeEnum.Adapter.class)
public enum ReleaseTypeEnum {
C("C"),
D("D"),
P("P"),
T("T");
private String value;
ReleaseTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ReleaseTypeEnum fromValue(String value) {
for (ReleaseTypeEnum b : ReleaseTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ReleaseTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ReleaseTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ReleaseTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_RELEASE_TYPE = "releaseType";
@SerializedName(SERIALIZED_NAME_RELEASE_TYPE)
private ReleaseTypeEnum releaseType;
/**
* Flags a transaction as a VIP order (based on merchant criteria). This field should not be sent for non-VIP orders.
*/
@JsonAdapter(VipCustomerEnum.Adapter.class)
public enum VipCustomerEnum {
Y("Y"),
N("N");
private String value;
VipCustomerEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static VipCustomerEnum fromValue(String value) {
for (VipCustomerEnum b : VipCustomerEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final VipCustomerEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public VipCustomerEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return VipCustomerEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_VIP_CUSTOMER = "vipCustomer";
@SerializedName(SERIALIZED_NAME_VIP_CUSTOMER)
private VipCustomerEnum vipCustomer;
public static final String SERIALIZED_NAME_SESSION_ID = "sessionId";
@SerializedName(SERIALIZED_NAME_SESSION_ID)
private String sessionId;
public static final String SERIALIZED_NAME_TERMINAL_STATE = "terminalState";
@SerializedName(SERIALIZED_NAME_TERMINAL_STATE)
private String terminalState;
public static final String SERIALIZED_NAME_TERMINAL_CITY = "terminalCity";
@SerializedName(SERIALIZED_NAME_TERMINAL_CITY)
private String terminalCity;
public static final String SERIALIZED_NAME_ACH_BILL_TO = "achBillTo";
@SerializedName(SERIALIZED_NAME_ACH_BILL_TO)
private TeleCheckAchPaymentMethodAchBillTo achBillTo;
public TeleCheckAchPaymentMethod() {
this.achType = this.getClass().getSimpleName();
}
public TeleCheckAchPaymentMethod achType(String achType) {
this.achType = achType;
return this;
}
/**
* ACH application type values will be one of either TeleCheckICAPaymentMethod or TeleCheckCBPPaymentMethod.
* @return achType
**/
@ApiModelProperty(example = "TeleCheckICAPaymentMethod", required = true, value = "ACH application type values will be one of either TeleCheckICAPaymentMethod or TeleCheckCBPPaymentMethod.")
public String getAchType() {
return achType;
}
public void setAchType(String achType) {
this.achType = achType;
}
public TeleCheckAchPaymentMethod routingNumber(String routingNumber) {
this.routingNumber = routingNumber;
return this;
}
/**
* Bank routing number.
* @return routingNumber
**/
@ApiModelProperty(example = "1283673312", required = true, value = "Bank routing number.")
public String getRoutingNumber() {
return routingNumber;
}
public void setRoutingNumber(String routingNumber) {
this.routingNumber = routingNumber;
}
public TeleCheckAchPaymentMethod accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Bank account number.
* @return accountNumber
**/
@ApiModelProperty(example = "2398649823984789", required = true, value = "Bank account number.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public TeleCheckAchPaymentMethod accountType(AccountTypeEnum accountType) {
this.accountType = accountType;
return this;
}
/**
* Identifies if the account type is checking or savings.
* @return accountType
**/
@ApiModelProperty(example = "C", required = true, value = "Identifies if the account type is checking or savings.")
public AccountTypeEnum getAccountType() {
return accountType;
}
public void setAccountType(AccountTypeEnum accountType) {
this.accountType = accountType;
}
public TeleCheckAchPaymentMethod checkNumber(String checkNumber) {
this.checkNumber = checkNumber;
return this;
}
/**
* Check number.
* @return checkNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "9878867880", value = "Check number.")
public String getCheckNumber() {
return checkNumber;
}
public void setCheckNumber(String checkNumber) {
this.checkNumber = checkNumber;
}
public TeleCheckAchPaymentMethod checkType(CheckTypeEnum checkType) {
this.checkType = checkType;
return this;
}
/**
* Identifies if the check type is personal or company.
* @return checkType
**/
@ApiModelProperty(example = "P", required = true, value = "Identifies if the check type is personal or company.")
public CheckTypeEnum getCheckType() {
return checkType;
}
public void setCheckType(CheckTypeEnum checkType) {
this.checkType = checkType;
}
public TeleCheckAchPaymentMethod productCode(String productCode) {
this.productCode = productCode;
return this;
}
/**
* Identifies the product code in the transaction.
* @return productCode
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "128367", value = "Identifies the product code in the transaction.")
public String getProductCode() {
return productCode;
}
public void setProductCode(String productCode) {
this.productCode = productCode;
}
public TeleCheckAchPaymentMethod manualIdInfo(IdInfo manualIdInfo) {
this.manualIdInfo = manualIdInfo;
return this;
}
/**
* Get manualIdInfo
* @return manualIdInfo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public IdInfo getManualIdInfo() {
return manualIdInfo;
}
public void setManualIdInfo(IdInfo manualIdInfo) {
this.manualIdInfo = manualIdInfo;
}
public TeleCheckAchPaymentMethod supplementIdInfo(IdInfo supplementIdInfo) {
this.supplementIdInfo = supplementIdInfo;
return this;
}
/**
* Get supplementIdInfo
* @return supplementIdInfo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public IdInfo getSupplementIdInfo() {
return supplementIdInfo;
}
public void setSupplementIdInfo(IdInfo supplementIdInfo) {
this.supplementIdInfo = supplementIdInfo;
}
public TeleCheckAchPaymentMethod agentId(String agentId) {
this.agentId = agentId;
return this;
}
/**
* Used to track the agent transaction activity.
* @return agentId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "RVK001", value = "Used to track the agent transaction activity.")
public String getAgentId() {
return agentId;
}
public void setAgentId(String agentId) {
this.agentId = agentId;
}
public TeleCheckAchPaymentMethod terminalId(String terminalId) {
this.terminalId = terminalId;
return this;
}
/**
* Identifies the register or lane number where the original sale transaction occurred.
* @return terminalId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1283673312", value = "Identifies the register or lane number where the original sale transaction occurred.")
public String getTerminalId() {
return terminalId;
}
public void setTerminalId(String terminalId) {
this.terminalId = terminalId;
}
public TeleCheckAchPaymentMethod registrationId(String registrationId) {
this.registrationId = registrationId;
return this;
}
/**
* Unique ID assigned by the merchant for the consumer (never recycled). It is an additional level of authentication. To use this feature, the merchant must work with TeleCheck Risk to discuss. Registration IDs must not be generated for an existing or returning consumer returns. The single registration ID must be unique per consumer.
* @return registrationId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "12345", value = "Unique ID assigned by the merchant for the consumer (never recycled). It is an additional level of authentication. To use this feature, the merchant must work with TeleCheck Risk to discuss. Registration IDs must not be generated for an existing or returning consumer returns. The single registration ID must be unique per consumer.")
public String getRegistrationId() {
return registrationId;
}
public void setRegistrationId(String registrationId) {
this.registrationId = registrationId;
}
public TeleCheckAchPaymentMethod registrationDate(LocalDate registrationDate) {
this.registrationDate = registrationDate;
return this;
}
/**
* Date the consumer originally registered in format MMDDYYYY.
* @return registrationDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Tue Oct 01 20:00:00 EDT 2019", value = "Date the consumer originally registered in format MMDDYYYY.")
public LocalDate getRegistrationDate() {
return registrationDate;
}
public void setRegistrationDate(LocalDate registrationDate) {
this.registrationDate = registrationDate;
}
public TeleCheckAchPaymentMethod releaseType(ReleaseTypeEnum releaseType) {
this.releaseType = releaseType;
return this;
}
/**
* Release type is used as a risk variable to gauge risk level when the merchant is releasing the purchased merchandise.
* @return releaseType
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "P", value = "Release type is used as a risk variable to gauge risk level when the merchant is releasing the purchased merchandise.")
public ReleaseTypeEnum getReleaseType() {
return releaseType;
}
public void setReleaseType(ReleaseTypeEnum releaseType) {
this.releaseType = releaseType;
}
public TeleCheckAchPaymentMethod vipCustomer(VipCustomerEnum vipCustomer) {
this.vipCustomer = vipCustomer;
return this;
}
/**
* Flags a transaction as a VIP order (based on merchant criteria). This field should not be sent for non-VIP orders.
* @return vipCustomer
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Y", value = "Flags a transaction as a VIP order (based on merchant criteria). This field should not be sent for non-VIP orders.")
public VipCustomerEnum getVipCustomer() {
return vipCustomer;
}
public void setVipCustomer(VipCustomerEnum vipCustomer) {
this.vipCustomer = vipCustomer;
}
public TeleCheckAchPaymentMethod sessionId(String sessionId) {
this.sessionId = sessionId;
return this;
}
/**
* Session identifier.
* @return sessionId
**/
@ApiModelProperty(example = "fb2e77d.47a0479900504cb3ab4a1f626d174d2d", required = true, value = "Session identifier.")
public String getSessionId() {
return sessionId;
}
public void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
public TeleCheckAchPaymentMethod terminalState(String terminalState) {
this.terminalState = terminalState;
return this;
}
/**
* Identifies the US state or territory where the original sale transaction occurred.
* @return terminalState
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "GA", value = "Identifies the US state or territory where the original sale transaction occurred.")
public String getTerminalState() {
return terminalState;
}
public void setTerminalState(String terminalState) {
this.terminalState = terminalState;
}
public TeleCheckAchPaymentMethod terminalCity(String terminalCity) {
this.terminalCity = terminalCity;
return this;
}
/**
* Identifies the city where the original sale transaction occurred.
* @return terminalCity
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Atlanta", value = "Identifies the city where the original sale transaction occurred.")
public String getTerminalCity() {
return terminalCity;
}
public void setTerminalCity(String terminalCity) {
this.terminalCity = terminalCity;
}
public TeleCheckAchPaymentMethod achBillTo(TeleCheckAchPaymentMethodAchBillTo achBillTo) {
this.achBillTo = achBillTo;
return this;
}
/**
* Get achBillTo
* @return achBillTo
**/
@ApiModelProperty(required = true, value = "")
public TeleCheckAchPaymentMethodAchBillTo getAchBillTo() {
return achBillTo;
}
public void setAchBillTo(TeleCheckAchPaymentMethodAchBillTo achBillTo) {
this.achBillTo = achBillTo;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TeleCheckAchPaymentMethod teleCheckAchPaymentMethod = (TeleCheckAchPaymentMethod) o;
return Objects.equals(this.achType, teleCheckAchPaymentMethod.achType) &&
Objects.equals(this.routingNumber, teleCheckAchPaymentMethod.routingNumber) &&
Objects.equals(this.accountNumber, teleCheckAchPaymentMethod.accountNumber) &&
Objects.equals(this.accountType, teleCheckAchPaymentMethod.accountType) &&
Objects.equals(this.checkNumber, teleCheckAchPaymentMethod.checkNumber) &&
Objects.equals(this.checkType, teleCheckAchPaymentMethod.checkType) &&
Objects.equals(this.productCode, teleCheckAchPaymentMethod.productCode) &&
Objects.equals(this.manualIdInfo, teleCheckAchPaymentMethod.manualIdInfo) &&
Objects.equals(this.supplementIdInfo, teleCheckAchPaymentMethod.supplementIdInfo) &&
Objects.equals(this.agentId, teleCheckAchPaymentMethod.agentId) &&
Objects.equals(this.terminalId, teleCheckAchPaymentMethod.terminalId) &&
Objects.equals(this.registrationId, teleCheckAchPaymentMethod.registrationId) &&
Objects.equals(this.registrationDate, teleCheckAchPaymentMethod.registrationDate) &&
Objects.equals(this.releaseType, teleCheckAchPaymentMethod.releaseType) &&
Objects.equals(this.vipCustomer, teleCheckAchPaymentMethod.vipCustomer) &&
Objects.equals(this.sessionId, teleCheckAchPaymentMethod.sessionId) &&
Objects.equals(this.terminalState, teleCheckAchPaymentMethod.terminalState) &&
Objects.equals(this.terminalCity, teleCheckAchPaymentMethod.terminalCity) &&
Objects.equals(this.achBillTo, teleCheckAchPaymentMethod.achBillTo);
}
@Override
public int hashCode() {
return Objects.hash(achType, routingNumber, accountNumber, accountType, checkNumber, checkType, productCode, manualIdInfo, supplementIdInfo, agentId, terminalId, registrationId, registrationDate, releaseType, vipCustomer, sessionId, terminalState, terminalCity, achBillTo);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TeleCheckAchPaymentMethod {\n");
sb.append(" achType: ").append(toIndentedString(achType)).append("\n");
sb.append(" routingNumber: ").append(toIndentedString(routingNumber)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" accountType: ").append(toIndentedString(accountType)).append("\n");
sb.append(" checkNumber: ").append(toIndentedString(checkNumber)).append("\n");
sb.append(" checkType: ").append(toIndentedString(checkType)).append("\n");
sb.append(" productCode: ").append(toIndentedString(productCode)).append("\n");
sb.append(" manualIdInfo: ").append(toIndentedString(manualIdInfo)).append("\n");
sb.append(" supplementIdInfo: ").append(toIndentedString(supplementIdInfo)).append("\n");
sb.append(" agentId: ").append(toIndentedString(agentId)).append("\n");
sb.append(" terminalId: ").append(toIndentedString(terminalId)).append("\n");
sb.append(" registrationId: ").append(toIndentedString(registrationId)).append("\n");
sb.append(" registrationDate: ").append(toIndentedString(registrationDate)).append("\n");
sb.append(" releaseType: ").append(toIndentedString(releaseType)).append("\n");
sb.append(" vipCustomer: ").append(toIndentedString(vipCustomer)).append("\n");
sb.append(" sessionId: ").append(toIndentedString(sessionId)).append("\n");
sb.append(" terminalState: ").append(toIndentedString(terminalState)).append("\n");
sb.append(" terminalCity: ").append(toIndentedString(terminalCity)).append("\n");
sb.append(" achBillTo: ").append(toIndentedString(achBillTo)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy