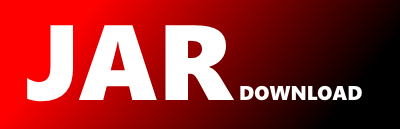
com.github.GBSEcom.model.TeleCheckAchPaymentMethodAchBillTo Maven / Gradle / Ivy
/*
* Payment Gateway API Specification.
* The documentation here is designed to provide all of the technical guidance required to consume and integrate with our APIs for payment processing. To learn more about our APIs please visit https://docs.firstdata.com/org/gateway.
*
* The version of the OpenAPI document: 21.2.0.20210406.001
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.github.GBSEcom.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Billing details for telecheck transactions.
*/
@ApiModel(description = "Billing details for telecheck transactions.")
public class TeleCheckAchPaymentMethodAchBillTo {
public static final String SERIALIZED_NAME_FIRST_NAME = "firstName";
@SerializedName(SERIALIZED_NAME_FIRST_NAME)
private String firstName;
public static final String SERIALIZED_NAME_LAST_NAME = "lastName";
@SerializedName(SERIALIZED_NAME_LAST_NAME)
private String lastName;
public static final String SERIALIZED_NAME_ADDRESS_ONE = "addressOne";
@SerializedName(SERIALIZED_NAME_ADDRESS_ONE)
private String addressOne;
public static final String SERIALIZED_NAME_ADDRESS_TWO = "addressTwo";
@SerializedName(SERIALIZED_NAME_ADDRESS_TWO)
private String addressTwo;
public static final String SERIALIZED_NAME_CITY = "city";
@SerializedName(SERIALIZED_NAME_CITY)
private String city;
public static final String SERIALIZED_NAME_STATE = "state";
@SerializedName(SERIALIZED_NAME_STATE)
private String state;
public static final String SERIALIZED_NAME_ZIP = "zip";
@SerializedName(SERIALIZED_NAME_ZIP)
private String zip;
public static final String SERIALIZED_NAME_PHONE = "phone";
@SerializedName(SERIALIZED_NAME_PHONE)
private String phone;
public static final String SERIALIZED_NAME_EMAIL = "email";
@SerializedName(SERIALIZED_NAME_EMAIL)
private String email;
public static final String SERIALIZED_NAME_COUNTRY_CODE = "countryCode";
@SerializedName(SERIALIZED_NAME_COUNTRY_CODE)
private String countryCode;
public TeleCheckAchPaymentMethodAchBillTo firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* Customer billing first name.
* @return firstName
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "R2-D2", value = "Customer billing first name.")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public TeleCheckAchPaymentMethodAchBillTo lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Customer billing last name.
* @return lastName
**/
@ApiModelProperty(example = "O'Calloway", required = true, value = "Customer billing last name.")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public TeleCheckAchPaymentMethodAchBillTo addressOne(String addressOne) {
this.addressOne = addressOne;
return this;
}
/**
* Customer billing address, first line.
* @return addressOne
**/
@ApiModelProperty(example = "1234 Line Ave S.", required = true, value = "Customer billing address, first line.")
public String getAddressOne() {
return addressOne;
}
public void setAddressOne(String addressOne) {
this.addressOne = addressOne;
}
public TeleCheckAchPaymentMethodAchBillTo addressTwo(String addressTwo) {
this.addressTwo = addressTwo;
return this;
}
/**
* Customer billing address, second line.
* @return addressTwo
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Ste 602", value = "Customer billing address, second line.")
public String getAddressTwo() {
return addressTwo;
}
public void setAddressTwo(String addressTwo) {
this.addressTwo = addressTwo;
}
public TeleCheckAchPaymentMethodAchBillTo city(String city) {
this.city = city;
return this;
}
/**
* Customer billing city.
* @return city
**/
@ApiModelProperty(example = "Atlanta", required = true, value = "Customer billing city.")
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public TeleCheckAchPaymentMethodAchBillTo state(String state) {
this.state = state;
return this;
}
/**
* Customer billing state.
* @return state
**/
@ApiModelProperty(example = "GA", required = true, value = "Customer billing state.")
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public TeleCheckAchPaymentMethodAchBillTo zip(String zip) {
this.zip = zip;
return this;
}
/**
* Customer billing zip code.
* @return zip
**/
@ApiModelProperty(example = "30040-1309", required = true, value = "Customer billing zip code.")
public String getZip() {
return zip;
}
public void setZip(String zip) {
this.zip = zip;
}
public TeleCheckAchPaymentMethodAchBillTo phone(String phone) {
this.phone = phone;
return this;
}
/**
* Customer billing phone number.
* @return phone
**/
@ApiModelProperty(example = "9973322990", required = true, value = "Customer billing phone number.")
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public TeleCheckAchPaymentMethodAchBillTo email(String email) {
this.email = email;
return this;
}
/**
* Customer billing email. Required if performing an ICA transaction.
* @return email
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[email protected]", value = "Customer billing email. Required if performing an ICA transaction.")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public TeleCheckAchPaymentMethodAchBillTo countryCode(String countryCode) {
this.countryCode = countryCode;
return this;
}
/**
* ISO country code. Required if performing an ICA transaction.
* @return countryCode
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "US", value = "ISO country code. Required if performing an ICA transaction.")
public String getCountryCode() {
return countryCode;
}
public void setCountryCode(String countryCode) {
this.countryCode = countryCode;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TeleCheckAchPaymentMethodAchBillTo teleCheckAchPaymentMethodAchBillTo = (TeleCheckAchPaymentMethodAchBillTo) o;
return Objects.equals(this.firstName, teleCheckAchPaymentMethodAchBillTo.firstName) &&
Objects.equals(this.lastName, teleCheckAchPaymentMethodAchBillTo.lastName) &&
Objects.equals(this.addressOne, teleCheckAchPaymentMethodAchBillTo.addressOne) &&
Objects.equals(this.addressTwo, teleCheckAchPaymentMethodAchBillTo.addressTwo) &&
Objects.equals(this.city, teleCheckAchPaymentMethodAchBillTo.city) &&
Objects.equals(this.state, teleCheckAchPaymentMethodAchBillTo.state) &&
Objects.equals(this.zip, teleCheckAchPaymentMethodAchBillTo.zip) &&
Objects.equals(this.phone, teleCheckAchPaymentMethodAchBillTo.phone) &&
Objects.equals(this.email, teleCheckAchPaymentMethodAchBillTo.email) &&
Objects.equals(this.countryCode, teleCheckAchPaymentMethodAchBillTo.countryCode);
}
@Override
public int hashCode() {
return Objects.hash(firstName, lastName, addressOne, addressTwo, city, state, zip, phone, email, countryCode);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TeleCheckAchPaymentMethodAchBillTo {\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" addressOne: ").append(toIndentedString(addressOne)).append("\n");
sb.append(" addressTwo: ").append(toIndentedString(addressTwo)).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" zip: ").append(toIndentedString(zip)).append("\n");
sb.append(" phone: ").append(toIndentedString(phone)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" countryCode: ").append(toIndentedString(countryCode)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy