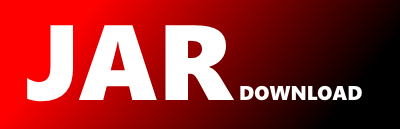
xdean.jex.extra.collection.LinkedMonotoneList Maven / Gradle / Ivy
The newest version!
package xdean.jex.extra.collection;
import java.util.AbstractList;
import java.util.Comparator;
import xdean.jex.extra.collection.LinkedList.Node;
/**
* A monotone increase list
*
* @author XDean
*
* @param
*/
public class LinkedMonotoneList extends AbstractList {
public enum MonoType {
/** When add an element, put it into the correct position **/
INSERT,
/**
* When add an element, link it to the last, and then delete as less as possible elements to
* make the list monotone again
**/
OVERWRITE;
}
private Comparator compartor;
private LinkedList list = new LinkedList<>();
private MonoType type;
public LinkedMonotoneList(Comparator comp, MonoType type) {
this.compartor = comp;
this.type = type;
}
@Override
public boolean add(E e) {
if (type == MonoType.INSERT) {
Node node = list.last;
while (compartor.compare(node.item, e) > 0) {
node = node.prev;
}
if (node == list.last) {
list.linkLast(e);
} else {
list.linkBefore(e, node.next);
}
return true;
} else if (type == MonoType.OVERWRITE) {
while (list.last != null && compartor.compare(list.last.item, e) > 0) {
list.unlink(list.last);
}
list.linkLast(e);
return true;
} else {
throw new UnsupportedOperationException();
}
}
@Override
public E get(int index) {
return list.get(index);
}
@Override
public E remove(int index) {
return list.remove(index);
}
@Override
public boolean remove(Object o) {
return list.remove(o);
}
@Override
public int size() {
return list.size;
}
@Override
public String toString() {
return "LinkedMonotoneList [type=" + type + ", list=" + list + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy