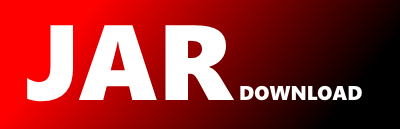
xdean.jex.extra.tryto.Try Maven / Gradle / Ivy
package xdean.jex.extra.tryto;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import xdean.jex.extra.function.ActionE0;
import xdean.jex.extra.function.FuncE0;
import xdean.jex.log.LogFactory;
/**
* Try pattern, similar to Optional (learn from Scala)
*
* @author [email protected]
*
*/
public abstract class Try{
/**
* Constructs a `Try` using a code as a supplier.
*/
public static Try to(FuncE0 code, ActionE0 onFinally) {
try {
return new Success<>(code.call());
} catch (Exception e) {
return new Failure<>(e);
} finally {
if (onFinally != null) {
try {
onFinally.call();
} catch (Exception e) {
LogFactory.from(Try.class).trace().log(e.getMessage(), e);
}
}
}
}
public static Try to(FuncE0 code) {
return Try.to(code, null);
}
public static Try to(ActionE0 code, ActionE0 onFinally) {
return Try.to(() -> {
code.call();
return null;
}, onFinally);
}
public static Try to(ActionE0 code) {
return Try.to(code, null);
}
public static Try of(T value) {
return new Success<>(value);
}
public static Try ofFailure(T value) {
return new Failure<>(value);
}
/**
* Returns `true` if the `Try` is a `Failure`, `false` otherwise.
*/
public abstract boolean isFailure();
/**
* Returns `true` if the `Try` is a `Success`, `false` otherwise.
*/
public abstract boolean isSuccess();
/**
* Returns the value from this `Success` or the given `default` argument if this is a `Failure`.
*
* ''Note:'': This will throw an exception if it is not a success and default throws an exception.
*/
public T getOrElse(Supplier defaultValue) {
return isSuccess() ? get() : defaultValue.get();
}
/**
* Returns the value from this `Success` or the given `default` argument if this is a `Failure`.
*
* ''Note:'': This will throw an exception if it is not a success and default throws an exception.
*/
public T getOrElse(T defaultValue) {
return isSuccess() ? get() : defaultValue;
}
/**
* Returns this `Try` if it's a `Success` or the given `default` argument if this is a `Failure`.
*/
public Try orElse(Supplier> defaultValue) {
try {
return isSuccess() ? this : defaultValue.get();
} catch (RuntimeException e) {
return new Failure<>(e);
}
}
/**
* Returns this `Try` if it's a `Success` or the given `default` argument if this is a `Failure`.
*/
public Try orElse(Try defaultValue) {
return isSuccess() ? this : defaultValue;
}
/**
* Returns the value from this `Success` or throws the exception if this is a `Failure`.
*/
public abstract T get();
/**
* Applies the given function `f` if this is a `Success`, otherwise returns `Unit` if this is a `Failure`.
*
* ''Note:'' If `f` throws, then this method may throw an exception.
*/
public abstract Try foreach(Consumer f);
public abstract Try onException(Consumer f);
/**
* Returns the given function applied to the value from this `Success` or returns this if this is a `Failure`.
*/
public abstract Try flatMap(Function> f);
/**
* Maps the given function to the value from this `Success` or returns this if this is a `Failure`.
*/
public abstract Try map(Function f);
/**
* Converts this to a `Failure` if the predicate is not satisfied.
*/
public abstract Try filter(Predicate p);
/**
* Applies the given function `f` if this is a `Failure`, otherwise returns this if this is a `Success`. This is like
* `flatMap` for the exception.
*/
public abstract Try recoverWith(Function> f);
/**
* Applies the given function `f` if this is a `Failure`, otherwise returns this if this is a `Success`. This is like
* map for the exception.
*/
public abstract Try recover(Function f);
/**
* Returns `None` if this is a `Failure` or a `Some` containing the value if this is a `Success`.
*/
public Optional toOptional() {
return isSuccess() ? Optional.ofNullable(get()) : Optional.empty();
}
/**
* Inverts this `Try`. If this is a `Failure`, returns its exception wrapped in a `Success`. If this is a `Success`,
* returns a `Failure` containing an `UnsupportedOperationException`.
*/
public abstract Try failed();
/**
* Completes this `Try` by applying the function `f` to this if this is of type `Failure`, or conversely, by applying
* `s` if this is a `Success`.
*/
public abstract Try transform(Function> s, Function> f);
}