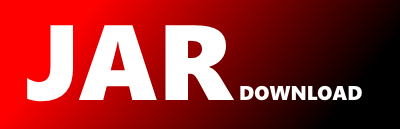
xdean.jex.util.cache.CacheUtil Maven / Gradle / Ivy
The newest version!
package xdean.jex.util.cache;
import java.lang.ref.WeakReference;
import java.util.Collections;
import java.util.Map;
import java.util.Optional;
import java.util.function.Supplier;
import com.google.common.collect.MapMaker;
@SuppressWarnings("unchecked")
public class CacheUtil {
private static final Map, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy