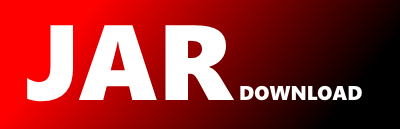
xdean.jex.util.task.If Maven / Gradle / Ivy
The newest version!
package xdean.jex.util.task;
import static xdean.jex.util.lang.ExceptionUtil.uncatch;
import java.util.NoSuchElementException;
import java.util.Optional;
import java.util.function.Supplier;
public final class If {
public static If that(boolean b) {
return new If<>(b);
}
private static final Object NULL_RESULT = new Object();
private final If parent;
private final boolean condition;
@SuppressWarnings("unchecked")
private T result = (T) NULL_RESULT;
public If(boolean condition) {
this.condition = condition;
this.parent = null;
}
public If(boolean condition, If parent) {
this.condition = condition;
this.parent = parent;
}
public If or(Supplier b) {
return new If<>(condition || b.get(), this);
}
public If and(Supplier b) {
return new If<>(condition && b.get(), this);
}
public If or(boolean b) {
return new If<>(condition || b, this);
}
public If and(boolean b) {
return new If<>(condition && b, this);
}
public If todo(Runnable r) {
if (condition) {
r.run();
}
return this;
}
public If tobe(T t) {
return tobe(() -> t);
}
public If tobe(Supplier s) {
if (condition) {
result = s.get();
}
return this;
}
public If ordo(Runnable r) {
if (!condition) {
r.run();
}
return this;
}
public If orbe(T t) {
return orbe(() -> t);
}
public If orbe(Supplier s) {
if (!condition) {
result = s.get();
}
return this;
}
public boolean condition() {
return condition;
}
public If end(boolean withResult) {
if (parent != null) {
if (withResult && result != NULL_RESULT) {
parent.result = result;
}
return parent;
} else {
throw new IllegalStateException("End more than if!");
}
}
public If end() {
return end(false);
}
/**
* Get the result.
*
* @return
* @throws NoSuchElementException If there is no result be set.
*/
public T result() throws NoSuchElementException {
if (result == NULL_RESULT) {
if (parent != null) {
return parent.result();
}
throw new NoSuchElementException("There is no result");
}
return result;
}
/**
* Get the result without exception.
* Note that this method don't distinguish no result and null result.
*
* @return
*/
public Optional safeResult() {
return Optional.ofNullable(uncatch(() -> result()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy