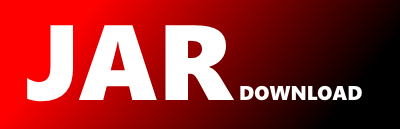
xdean.annotation.MethodRef Maven / Gradle / Ivy
The newest version!
package xdean.annotation;
import static java.lang.annotation.ElementType.METHOD;
import java.lang.annotation.Annotation;
import java.lang.annotation.Documented;
import java.lang.annotation.Target;
/**
* Annotated on annotation's attribute to indicate that it is referenced to a method.
*
* @see Type
* @author XDean
*/
@Documented
@Target(METHOD)
public @interface MethodRef {
public enum Type {
/**
* Annotated on a String attribute, its value will be parsed to class name and method name.
*
* Example:
*
*
*
//define
@interface UseAll {
@MethodRef //default type is All
String value();
}
//usage
@UseAll("java.lang.String:length")
void func();
*
*
* @see MethodRef#splitor()
*/
ALL,
/**
* Only used with {@link Type#METHOD}
*
* @see Type#METHOD
*/
CLASS,
/**
* Annotated on a String attribute, it has following modes:
*
* - Use with another attribute with type={@link Type#CLASS}(also can set a parent class), reference method from
* class by the other attribute's value
* - Use with {@link MethodRef#defaultClass()}, reference method from the determined class
* - Use with {@link MethodRef#parentClass()}, reference method from class by its EnclosingElement(usually a
* class)'s annotation's value
*
*
* Example:
* 1. Note that if you use Class and Method, there must have and only have 2 attribute with @{@link MethodRef}.
* And the Class attribute can also set a parent class, if the class value is {@code void.class}, the parent
* annotation value will be used.
*
*
*
//define
@interface UseClassAndMethod {
@MethodRef(type = Type.CLASS)
Class<? extends Number> type();
@MethodRef(type = Type.METHOD)
String method();
}
//usage
@UseClassAndMethod(type = Integer.class, method = "intValue")
void func();
*
*
*
*
//define
@interface UseClassAndMethodWithParent {
@interface Parent {
Class<?> value();
}
@MethodRef(type = Type.CLASS, parentClass = Parent.class)
Class<?> type() default void.class;
@MethodRef(type = Type.METHOD)
String method();
}
//usage
@Parent(Integer.class)
class Bar{
@UseClassAndMethodWithParent(method = "intValue")
void func();
}
*
*
* 2.
*
*
*
//define
@interface UseDefaultClass {
@MethodRef(type = Type.METHOD, defaultClass = String.class)
String method();
}
//usage
@UseDefaultClass(method = "length")
void func();
*
*
* 3. Note that the parent annotation must have a Class attribute named 'value'.
*
*
*
//define
@interface UseParentClass {
@interface Parent {
Class<?> value();
}
@MethodRef(type = Type.METHOD, parentClass = Parent.class)
String method();
}
//usage
@Parent(String.class)
class Bar{
@UseParentClass(method = "length")
void func();
}
*
*/
METHOD
}
/**
* @see Type
* @return type
*/
Type type() default Type.ALL;
/**
* If using {@link Type#ALL}, the attribute value will be split by splitor.
* For example: "java.lang.String:length" with splitor ':'.
*
* @see Type#METHOD
* @return splitor char
*/
char splitor() default ':';
/**
* @see Type#METHOD
* @return parent class
*/
Class extends Annotation> parentClass() default MethodRef.class;
/**
* @see Type#METHOD
* @return default class
*/
Class> defaultClass() default void.class;
/**
* @see Type#METHOD
* @return if need find method in enclosing element
*/
boolean findInEnclosing() default false;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy