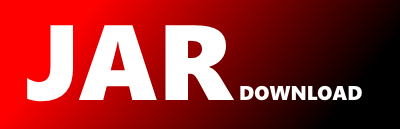
com.intellij.compiler.ModuleCompilerUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compiler-openapi Show documentation
Show all versions of compiler-openapi Show documentation
A packaging of the IntelliJ Community Edition compiler-openapi library.
This is release number 1 of trunk branch 142.
The newest version!
/*
* Copyright 2000-2014 JetBrains s.r.o.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.intellij.compiler;
import com.intellij.openapi.application.Application;
import com.intellij.openapi.application.ApplicationManager;
import com.intellij.openapi.diagnostic.Logger;
import com.intellij.openapi.module.Module;
import com.intellij.openapi.module.ModuleManager;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.roots.*;
import com.intellij.openapi.roots.ui.configuration.DefaultModulesProvider;
import com.intellij.openapi.util.Condition;
import com.intellij.openapi.util.Couple;
import com.intellij.util.Chunk;
import com.intellij.util.Processor;
import com.intellij.util.containers.ContainerUtil;
import com.intellij.util.graph.*;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.*;
/**
* @author dsl
*/
public final class ModuleCompilerUtil {
private static final Logger LOG = Logger.getInstance("#com.intellij.compiler.ModuleCompilerUtil");
private ModuleCompilerUtil() { }
public static Module[] getDependencies(Module module) {
return ModuleRootManager.getInstance(module).getDependencies();
}
public static Graph createModuleGraph(final Module[] modules) {
return GraphGenerator.create(CachingSemiGraph.create(new GraphGenerator.SemiGraph() {
public Collection getNodes() {
return Arrays.asList(modules);
}
public Iterator getIn(Module module) {
return Arrays.asList(getDependencies(module)).iterator();
}
}));
}
public static List> getSortedModuleChunks(Project project, List modules) {
final Module[] allModules = ModuleManager.getInstance(project).getModules();
final List> chunks = getSortedChunks(createModuleGraph(allModules));
final Set modulesSet = new HashSet(modules);
// leave only those chunks that contain at least one module from modules
for (Iterator> it = chunks.iterator(); it.hasNext();) {
final Chunk chunk = it.next();
if (!ContainerUtil.intersects(chunk.getNodes(), modulesSet)) {
it.remove();
}
}
return chunks;
}
public static List> getSortedChunks(final Graph graph) {
final Graph> chunkGraph = toChunkGraph(graph);
final List> chunks = new ArrayList>(chunkGraph.getNodes().size());
for (final Chunk chunk : chunkGraph.getNodes()) {
chunks.add(chunk);
}
DFSTBuilder> builder = new DFSTBuilder>(chunkGraph);
if (!builder.isAcyclic()) {
LOG.error("Acyclic graph expected");
return null;
}
Collections.sort(chunks, builder.comparator());
return chunks;
}
public static Graph> toChunkGraph(final Graph graph) {
return GraphAlgorithms.getInstance().computeSCCGraph(graph);
}
public static void sortModules(final Project project, final List modules) {
final Application application = ApplicationManager.getApplication();
Runnable sort = new Runnable() {
public void run() {
Comparator comparator = ModuleManager.getInstance(project).moduleDependencyComparator();
Collections.sort(modules, comparator);
}
};
if (application.isDispatchThread()) {
sort.run();
}
else {
application.runReadAction(sort);
}
}
public static GraphGenerator createGraphGenerator(final Map models) {
return GraphGenerator.create(CachingSemiGraph.create(new GraphGenerator.SemiGraph() {
public Collection getNodes() {
return models.values();
}
public Iterator getIn(final ModuleRootModel model) {
final List dependencies = new ArrayList();
model.orderEntries().compileOnly().forEachModule(new Processor() {
@Override
public boolean process(Module module) {
T depModel = models.get(module);
if (depModel != null) {
dependencies.add(depModel);
}
return true;
}
});
return dependencies.iterator();
}
}));
}
/**
* @return pair of modules which become circular after adding dependency, or null if all remains OK
*/
@Nullable
public static Couple addingDependencyFormsCircularity(final Module currentModule, Module toDependOn) {
assert currentModule != toDependOn;
// whatsa lotsa of @^%$ codes-a!
final Map models = new LinkedHashMap();
Project project = currentModule.getProject();
for (Module module : ModuleManager.getInstance(project).getModules()) {
ModifiableRootModel model = ModuleRootManager.getInstance(module).getModifiableModel();
models.put(module, model);
}
ModifiableRootModel currentModel = models.get(currentModule);
ModifiableRootModel toDependOnModel = models.get(toDependOn);
Collection> nodesBefore = buildChunks(models);
for (Chunk chunk : nodesBefore) {
if (chunk.containsNode(toDependOnModel) && chunk.containsNode(currentModel)) return null; // they circular already
}
try {
currentModel.addModuleOrderEntry(toDependOn);
Collection> nodesAfter = buildChunks(models);
for (Chunk chunk : nodesAfter) {
if (chunk.containsNode(toDependOnModel) && chunk.containsNode(currentModel)) {
Iterator nodes = chunk.getNodes().iterator();
return Couple.of(nodes.next().getModule(), nodes.next().getModule());
}
}
}
finally {
for (ModifiableRootModel model : models.values()) {
model.dispose();
}
}
return null;
}
public static Collection> buildChunks(final Map models) {
return toChunkGraph(createGraphGenerator(models)).getNodes();
}
public static List> getCyclicDependencies(@NotNull Project project, @NotNull List modules) {
Graph graph = createModuleSourceDependenciesGraph(new DefaultModulesProvider(project));
Collection> chunks = GraphAlgorithms.getInstance().computeStronglyConnectedComponents(graph);
final Set modulesSet = new HashSet(modules);
return ContainerUtil.filter(chunks, new Condition>() {
@Override
public boolean value(Chunk chunk) {
for (ModuleSourceSet sourceSet : chunk.getNodes()) {
if (modulesSet.contains(sourceSet.getModule())) {
return true;
}
}
return false;
}
});
}
public static Graph createModuleSourceDependenciesGraph(final RootModelProvider provider) {
return GraphGenerator.create(new CachingSemiGraph(new GraphGenerator.SemiGraph() {
@Override
public Collection getNodes() {
Module[] modules = provider.getModules();
List result = new ArrayList(modules.length * 2);
for (Module module : modules) {
result.add(new ModuleSourceSet(module, ModuleSourceSet.Type.PRODUCTION));
result.add(new ModuleSourceSet(module, ModuleSourceSet.Type.TEST));
}
return result;
}
@Override
public Iterator getIn(final ModuleSourceSet n) {
ModuleRootModel model = provider.getRootModel(n.getModule());
OrderEnumerator enumerator = model.orderEntries().compileOnly();
if (n.getType() == ModuleSourceSet.Type.PRODUCTION) {
enumerator = enumerator.productionOnly();
}
final List deps = new ArrayList();
enumerator.forEachModule(new Processor() {
@Override
public boolean process(Module module) {
deps.add(new ModuleSourceSet(module, n.getType()));
return true;
}
});
if (n.getType() == ModuleSourceSet.Type.TEST) {
deps.add(new ModuleSourceSet(n.getModule(), ModuleSourceSet.Type.PRODUCTION));
}
return deps.iterator();
}
}));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy