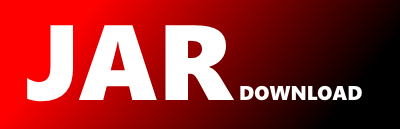
com.intellij.packaging.elements.CompositePackagingElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compiler-openapi Show documentation
Show all versions of compiler-openapi Show documentation
A packaging of the IntelliJ Community Edition compiler-openapi library.
This is release number 1 of trunk branch 142.
The newest version!
/*
* Copyright 2000-2009 JetBrains s.r.o.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.intellij.packaging.elements;
import com.intellij.compiler.ant.Generator;
import com.intellij.packaging.artifacts.ArtifactType;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
/**
* @author nik
*/
public abstract class CompositePackagingElement extends PackagingElement implements RenameablePackagingElement {
private final List> myChildren = new ArrayList>();
private List> myUnmodifiableChildren;
protected CompositePackagingElement(PackagingElementType type) {
super(type);
}
public > T addOrFindChild(@NotNull T child) {
for (PackagingElement> element : myChildren) {
if (element.isEqualTo(child)) {
if (element instanceof CompositePackagingElement) {
final List> children = ((CompositePackagingElement>)child).getChildren();
((CompositePackagingElement>)element).addOrFindChildren(children);
}
//noinspection unchecked
return (T) element;
}
}
myChildren.add(child);
return child;
}
public void addFirstChild(@NotNull PackagingElement> child) {
myChildren.add(0, child);
for (int i = 1; i < myChildren.size(); i++) {
PackagingElement> element = myChildren.get(i);
if (element.isEqualTo(child)) {
if (element instanceof CompositePackagingElement>) {
((CompositePackagingElement>)child).addOrFindChildren(((CompositePackagingElement>)element).getChildren());
}
myChildren.remove(i);
break;
}
}
}
public List extends PackagingElement>> addOrFindChildren(Collection extends PackagingElement>> children) {
List> added = new ArrayList>();
for (PackagingElement> child : children) {
added.add(addOrFindChild(child));
}
return added;
}
@Nullable
public PackagingElement> moveChild(int index, int direction) {
int target = index + direction;
if (0 <= index && index < myChildren.size() && 0 <= target && target < myChildren.size()) {
final PackagingElement> element1 = myChildren.get(index);
final PackagingElement> element2 = myChildren.get(target);
myChildren.set(index, element2);
myChildren.set(target, element1);
return element1;
}
return null;
}
public void removeChild(@NotNull PackagingElement> child) {
myChildren.remove(child);
}
public void removeChildren(@NotNull Collection extends PackagingElement>> children) {
myChildren.removeAll(children);
}
@NotNull
public List> getChildren() {
if (myUnmodifiableChildren == null) {
myUnmodifiableChildren = Collections.unmodifiableList(myChildren);
}
return myUnmodifiableChildren;
}
public boolean canBeRenamed() {
return true;
}
protected List extends Generator> computeChildrenGenerators(PackagingElementResolvingContext resolvingContext,
final AntCopyInstructionCreator copyInstructionCreator,
final ArtifactAntGenerationContext generationContext, ArtifactType artifactType) {
final List generators = new ArrayList();
for (PackagingElement> child : myChildren) {
generators.addAll(child.computeAntInstructions(resolvingContext, copyInstructionCreator, generationContext, artifactType));
}
return generators;
}
public void removeAllChildren() {
myChildren.clear();
}
@Nullable
public CompositePackagingElement> findCompositeChild(@NotNull String name) {
for (PackagingElement> child : myChildren) {
if (child instanceof CompositePackagingElement && name.equals(((CompositePackagingElement)child).getName())) {
return (CompositePackagingElement)child;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy