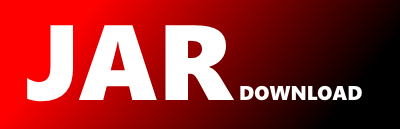
com.intellij.util.graph.impl.CycleFinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of platform-impl Show documentation
Show all versions of platform-impl Show documentation
A packaging of the IntelliJ Community Edition platform-impl library.
This is release number 1 of trunk branch 142.
The newest version!
/*
* Copyright 2000-2009 JetBrains s.r.o.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.intellij.util.graph.impl;
import com.intellij.util.graph.Graph;
import org.jetbrains.annotations.NotNull;
import java.util.*;
/**
* User: anna
* Date: Feb 13, 2005
*/
public class CycleFinder {
private final Graph myGraph;
public CycleFinder(Graph graph) {
myGraph = graph;
}
@NotNull
public Set> getNodeCycles(final Node node){
final Set> result = new HashSet>();
final Graph graphWithoutNode = new Graph() {
public Collection getNodes() {
final Collection nodes = myGraph.getNodes();
nodes.remove(node);
return nodes;
}
public Iterator getIn(final Node n) {
final HashSet nodes = new HashSet();
final Iterator in = myGraph.getIn(n);
while (in.hasNext()) {
nodes.add(in.next());
}
nodes.remove(node);
return nodes.iterator();
}
public Iterator getOut(final Node n) {
final HashSet nodes = new HashSet();
final Iterator out = myGraph.getOut(n);
while (out.hasNext()) {
nodes.add(out.next());
}
nodes.remove(node);
return nodes.iterator();
}
};
final HashSet inNodes = new HashSet();
final Iterator in = myGraph.getIn(node);
while (in.hasNext()) {
inNodes.add(in.next());
}
final HashSet outNodes = new HashSet();
final Iterator out = myGraph.getOut(node);
while (out.hasNext()) {
outNodes.add(out.next());
}
final HashSet retainNodes = new HashSet(inNodes);
retainNodes.retainAll(outNodes);
for (Node node1 : retainNodes) {
ArrayList oneNodeCycle = new ArrayList();
oneNodeCycle.add(node1);
oneNodeCycle.add(node);
result.add(oneNodeCycle);
}
inNodes.removeAll(retainNodes);
outNodes.removeAll(retainNodes);
ShortestPathFinder finder = new ShortestPathFinder(graphWithoutNode);
for (Node fromNode : outNodes) {
for (Node toNode : inNodes) {
final List shortestPath = finder.findPath(fromNode, toNode);
if (shortestPath != null) {
ArrayList path = new ArrayList();
path.addAll(shortestPath);
path.add(node);
result.add(path);
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy