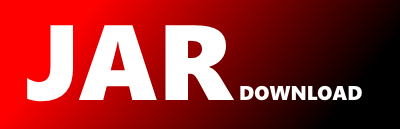
afester.javafx.examples.table.map.TableExample Maven / Gradle / Ivy
package afester.javafx.examples.table.map;
import java.util.HashMap;
import java.util.Map;
import afester.javafx.examples.Example;
import javafx.application.Application;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.MapValueFactory;
import javafx.scene.layout.Pane;
import javafx.stage.Stage;
/**
* This is a standard TableView example based on http://docs.oracle.com/javafx/2/ui_controls/table-view.htm
* It uses a Map as the data model.
*/
@Example(desc = "Map based Table",
cat = "Basic JavaFX")
public class TableExample extends Application {
public static void main(String[] args) {
launch(args);
}
public void run() {
start(new Stage());
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("JavaFX Table example");
TableView
© 2015 - 2025 Weber Informatics LLC | Privacy Policy