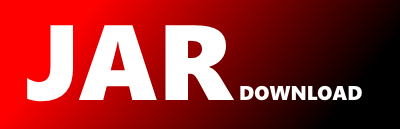
afester.javafx.examples.table.simple.TableExample Maven / Gradle / Ivy
package afester.javafx.examples.table.simple;
import afester.javafx.components.LiveTextFieldTableCell;
import afester.javafx.examples.Example;
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableColumn.CellEditEvent;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.CheckBoxTableCell;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.control.cell.TextFieldTableCell;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
/**
* This is a standard TableView example based on http://docs.oracle.com/javafx/2/ui_controls/table-view.htm
* In addition it contains one row where the cells are live editable
*/
@Example(desc = "Live editable Table",
cat = "FranzXaver")
public class TableExample extends Application {
private final ObservableList data =
FXCollections.observableArrayList(
new TableRow("Jacob", "Smith", "[email protected]"),
new TableRow("Isabella", "Johnson", "[email protected]"),
new TableRow("Ethan", "Williams", "[email protected]"),
new TableRow("Emma", "Jones", "[email protected]", true, "Hello World"),
new TableRow("Michael", "Brown", "[email protected]")
);
public static void main(String[] args) {
launch(args);
}
public void run() {
start(new Stage());
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("JavaFX Table example");
TableView table = new TableView<>();
table.setEditable(true);
table.setColumnResizePolicy(TableView.CONSTRAINED_RESIZE_POLICY);
TableColumn firstNameCol = new TableColumn<>("First Name");
firstNameCol.setCellValueFactory(
new PropertyValueFactory("firstName")
);
firstNameCol.setCellFactory(TextFieldTableCell.forTableColumn());
firstNameCol.setOnEditCommit(
new EventHandler>() {
@Override
public void handle(CellEditEvent t) {
String newValue = t.getNewValue();
int rowIdx = t.getTablePosition().getRow();
TableRow row = t.getTableView().getItems().get(rowIdx);
row.setFirstName(newValue);
}
}
);
TableColumn lastNameCol = new TableColumn<>("Last Name");
lastNameCol.setCellValueFactory(
new PropertyValueFactory("lastName")
);
TableColumn emailCol = new TableColumn<>("Email");
emailCol.setCellValueFactory(
new PropertyValueFactory("email")
);
TableColumn flagCol = new TableColumn<>("Flag");
flagCol.setCellFactory(CheckBoxTableCell.forTableColumn((TableColumn)null));
flagCol.setCellValueFactory(
new PropertyValueFactory("flag")
);
TableColumn inlineEditCol = new TableColumn<>("Edit");
inlineEditCol.setCellFactory(LiveTextFieldTableCell.forTableColumn());
// sets the obervable factory for the cell value.
// In the cell renderer, the corresponding observable can be retrieved through
// getTableColumn().getCellObservableValue(getIndex());
inlineEditCol.setCellValueFactory(
new PropertyValueFactory("comment")
);
table.getColumns().addAll(firstNameCol, lastNameCol, emailCol, flagCol, inlineEditCol);
// set data for the table
table.setItems(data);
VBox layout = new VBox();
layout.getChildren().add(table);
Button dumpIt = new Button("Dump");
dumpIt.setOnAction(e -> {
for (TableRow row : data) {
System.err.println(row.toString());
}
});
layout.getChildren().add(dumpIt);
// show the generated scene graph
Scene scene = new Scene(layout);
scene.getStylesheets().add("/afester/javafx/examples/table/simple/tableview.css");
primaryStage.setScene(scene);
primaryStage.show();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy