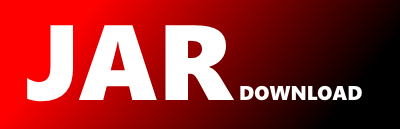
org.swaggertools.demo.client.PetsClient Maven / Gradle / Ivy
package org.swaggertools.demo.client;
import com.fasterxml.jackson.core.type.TypeReference;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Long;
import java.lang.String;
import java.util.List;
import java.util.Map;
import org.apache.http.impl.client.CloseableHttpClient;
import org.swaggertools.demo.model.Pet;
public class PetsClient extends BaseClient {
public PetsClient(CloseableHttpClient closeableHttpClient) {
super(closeableHttpClient);
}
public PetsClient(CloseableHttpClient closeableHttpClient, String basePath) {
super(closeableHttpClient, basePath);
}
public PetsClient(CloseableHttpClient closeableHttpClient, String basePath,
Map> headers) {
super(closeableHttpClient, basePath, headers);
}
public List listPets(Integer limit) {
TypeReference> typeRef = new TypeReference>(){};
return invokeAPI("/pets", "GET", createUrlVariables(), createQueryParameters("limit", limit), null, typeRef);
}
public Pet createPet(Pet requestBody) {
TypeReference typeRef = new TypeReference(){};
return invokeAPI("/pets", "POST", createUrlVariables(), createQueryParameters(), requestBody, typeRef);
}
public Pet getPetById(Long petId, Boolean details) {
TypeReference typeRef = new TypeReference(){};
return invokeAPI("/pets/{petId}", "GET", createUrlVariables("petId", petId), createQueryParameters("details", details), null, typeRef);
}
public void updatePet(Long petId, Pet requestBody) {
TypeReference typeRef = VOID;
invokeAPI("/pets/{petId}", "PUT", createUrlVariables("petId", petId), createQueryParameters(), requestBody, typeRef);
}
public void deletePetById(Long petId) {
TypeReference typeRef = VOID;
invokeAPI("/pets/{petId}", "DELETE", createUrlVariables("petId", petId), createQueryParameters(), null, typeRef);
}
public String getPetEvents(Long petId) {
TypeReference typeRef = new TypeReference(){};
return invokeAPI("/pets/{petId}/events", "GET", createUrlVariables("petId", petId), createQueryParameters(), null, typeRef);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy