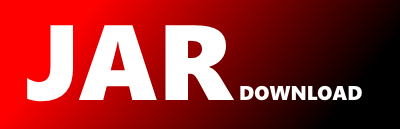
com.github.aidensuen.mongo.spring.mongodao.MongoDaoFactoryBean Maven / Gradle / Ivy
package com.github.aidensuen.mongo.spring.mongodao;
import com.github.aidensuen.mongo.core.MongoDaoRepository;
import com.github.aidensuen.mongo.session.Configuration;
import com.github.aidensuen.mongo.spring.support.MongoDaoSupport;
import org.springframework.beans.factory.FactoryBean;
import static org.springframework.util.Assert.notNull;
public class MongoDaoFactoryBean extends MongoDaoSupport implements FactoryBean {
private boolean addToConfig = true;
private Class mongoDaoInterface;
private MongoDaoRepository mongoDaoRepository;
public MongoDaoFactoryBean() {
}
public MongoDaoFactoryBean(Class mongoDaoInterface) {
this.mongoDaoInterface = mongoDaoInterface;
}
@Override
protected void checkDaoConfig() {
super.checkDaoConfig();
notNull(this.mongoDaoInterface, "Property 'mongoDaoInterface' is required");
Configuration configuration = this.getMongoSession().getConfiguration();
if (this.addToConfig && !configuration.hasMongoDao(this.mongoDaoInterface)) {
try {
configuration.addMongoDao(this.mongoDaoInterface);
} catch (Exception e) {
logger.error("Error while adding the dao '" + this.mongoDaoInterface + "' to configuration.", e);
throw new IllegalArgumentException(e);
}
}
// auto register generic MongoDao
if (configuration.hasMongoDao(this.mongoDaoInterface) && mongoDaoInterface != null && mongoDaoRepository.isExtendCommonMongoDao(this.mongoDaoInterface)) {
mongoDaoRepository.processConfiguration(this.getMongoSession().getConfiguration(), this.mongoDaoInterface);
}
}
@Override
public T getObject() throws Exception {
return (T) getMongoSession().getMongoDao(this.mongoDaoInterface);
}
@Override
public Class getObjectType() {
return this.mongoDaoInterface;
}
@Override
public boolean isSingleton() {
return true;
}
public MongoDaoRepository getMongoDaoRepository() {
return mongoDaoRepository;
}
public void setMongoDaoRepository(MongoDaoRepository mongoDaoRepository) {
this.mongoDaoRepository = mongoDaoRepository;
}
public void setAddToConfig(boolean addToConfig) {
this.addToConfig = addToConfig;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy