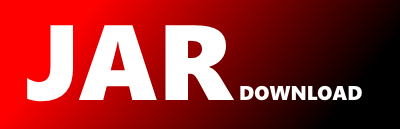
com.feinik.csv.CsvUtil Maven / Gradle / Ivy
package com.feinik.csv;
import com.csvreader.CsvReader;
import com.csvreader.CsvWriter;
import com.feinik.csv.analysis.CsvDataBuilder;
import com.feinik.csv.context.CsvContext;
import com.feinik.csv.event.CsvListener;
import com.feinik.csv.exception.CsvUtilException;
import java.io.InputStream;
import java.nio.charset.Charset;
import java.util.List;
/**
* CSV is read and written through the tool
*
* @author Feinik
*/
public class CsvUtil {
private static final int DEFAULT_SKIP_ROW = 1;
/**
* Write the template object to CSV
* @param writer get by CsvFactory
* @param writeData write data
* @param containHead whether to write to the head line
* @param
* @return
*/
public static boolean write(CsvWriter writer, List writeData, boolean containHead) {
return write(writer, writeData, containHead, null);
}
/**
* Write the template object to CSV
* @param writer get by CsvFactory
* @param writeData write data
* @param containHead whether to write to the head line
* @param context can init handle CsvWriter or CsvReader
* @param
* @return
*/
public static boolean write(CsvWriter writer, List writeData,
boolean containHead, CsvContext context) {
CsvDataBuilder builder = new CsvDataBuilder(context);
boolean result = false;
try {
builder.write(writer, containHead, writeData);
result = true;
} catch (Exception e) {
throw new CsvUtilException(e);
} finally {
return result;
}
}
/**
* Read small rows of data from CSV
* @param filePath read file path
* @param cls
* @param skipRow Skip the x line read
* @param charset
* @param
* @return result data
*/
public static List read(String filePath, Class cls, int skipRow, Charset charset) throws Exception {
final CsvReader reader = CsvFactory.getReader(filePath, charset);
return readContext(reader, cls, skipRow, null);
}
/**
* Read small rows of data from CSV, default skip head line
* @param filePath read file path
* @param cls
* @param charset
* @param
* @return result data
*/
public static List read(String filePath, Class cls, Charset charset) throws Exception {
final CsvReader reader = CsvFactory.getReader(filePath, charset);
return readContext(reader, cls, DEFAULT_SKIP_ROW, null);
}
/**
* Read small rows of data from CSV, with context
* @param filePath read file path
* @param cls
* @param skipRow Skip the x line read
* @param charset
* @param context can init handle CsvWriter or CsvReader
* @param
* @return
*/
public static List read(String filePath, Class cls,
int skipRow, Charset charset, CsvContext context) throws Exception {
final CsvReader reader = CsvFactory.getReader(filePath, charset);
return readContext(reader, cls, skipRow, context);
}
/**
* Read small rows of data from CSV, with context
* @param filePath read file path
* @param cls
* @param delimiter field separator
* @param skipRow Skip the x line read
* @param charset
* @param context can init handle CsvWriter or CsvReader
* @param
* @return
* @throws Exception
*/
public static List read(String filePath, Class cls, char delimiter,
int skipRow, Charset charset, CsvContext context) throws Exception {
final CsvReader reader = CsvFactory.getReader(filePath, delimiter, charset);
return readContext(reader, cls, skipRow, context);
}
/**
* Read small rows of data from CSV, with context
* @param is
* @param cls
* @param skipRow Skip the x line read
* @param charset
* @param context can init handle CsvWriter or CsvReader
* @param
* @return
* @throws Exception
*/
public static List read(InputStream is, Class cls,
int skipRow, Charset charset, CsvContext context) throws Exception {
final CsvReader reader = CsvFactory.getReader(is, charset);
return readContext(reader, cls, skipRow, context);
}
/**
* Read small rows of data from CSV, with context
* @param is
* @param cls
* @param delimiter field separator
* @param skipRow Skip the x line read
* @param charset
* @param context
* @param
* @return
* @throws Exception
*/
public static List read(InputStream is, Class cls, char delimiter,
int skipRow, Charset charset, CsvContext context) throws Exception {
final CsvReader reader = CsvFactory.getReader(is, delimiter, charset);
return readContext(reader, cls, skipRow, context);
}
/**
* Read small rows of data from CSV, with context.
* default skip head line
* @param filePath read file path
* @param cls
* @param charset
* @param context Can init CsvWriter or CsvReader
* @param
* @return
*/
public static List read(String filePath, Class cls,
Charset charset, CsvContext context) throws Exception {
final CsvReader reader = CsvFactory.getReader(filePath, charset);
return readContext(reader, cls, DEFAULT_SKIP_ROW, context);
}
/**
* Read the large CSV file
* @param filePath read file path
* @param cls
* @param skipRow Skip the x line read
* @param charset
* @param listener Synchronous call per read line
* @param
*/
public static void readOnListener(String filePath, Class cls,
int skipRow, Charset charset,
CsvListener listener) throws Exception {
final CsvReader reader = CsvFactory.getReader(filePath, charset);
readOnListenerAndWithContext(reader, cls, skipRow, null, listener);
}
/**
* Read the large CSV file
* @param filePath
* @param cls
* @param delimiter
* @param skipRow
* @param charset
* @param listener
* @param
* @throws Exception
*/
public static void readOnListener(String filePath, Class cls,
char delimiter,
int skipRow, Charset charset,
CsvListener listener) throws Exception {
final CsvReader reader = CsvFactory.getReader(filePath, delimiter, charset);
readOnListenerAndWithContext(reader, cls, skipRow, null, listener);
}
/**
* Read the large CSV file, default skip head line
* @param filePath read file path
* @param cls
* @param charset
* @param listener Synchronous call per read line
* @param
*/
public static void readOnListener(String filePath, Class cls,
Charset charset,
CsvListener listener) throws Exception {
final CsvReader reader = CsvFactory.getReader(filePath, charset);
readOnListenerAndWithContext(reader, cls, DEFAULT_SKIP_ROW, null, listener);
}
/**
* Read the large CSV file
* @param filePath read file path
* @param cls
* @param skipRow Skip the x line read
* @param charset
* @param context Can init CsvWriter or CsvReader
* @param listener Synchronous call per read line
* @param
*/
public static void readOnListener(String filePath, Class cls,
int skipRow, Charset charset,
CsvContext context,
CsvListener listener) throws Exception {
final CsvReader reader = CsvFactory.getReader(filePath, charset);
readOnListenerAndWithContext(reader, cls, skipRow, context, listener);
}
/**
* Read the large CSV file
* @param is
* @param cls
* @param skipRow
* @param charset
* @param context
* @param listener
* @param
*/
public static void readOnListener(InputStream is, Class cls,
int skipRow, Charset charset,
CsvContext context,
CsvListener listener) throws Exception {
final CsvReader reader = CsvFactory.getReader(is, charset);
readOnListenerAndWithContext(reader, cls, skipRow, context, listener);
}
/**
* Read the large CSV file
* @param is
* @param cls
* @param delimiter
* @param skipRow
* @param charset
* @param context
* @param listener
* @param
* @throws Exception
*/
public static void readOnListener(InputStream is, Class cls, char delimiter,
int skipRow, Charset charset,
CsvContext context,
CsvListener listener) throws Exception {
final CsvReader reader = CsvFactory.getReader(is, delimiter, charset);
readOnListenerAndWithContext(reader, cls, skipRow, context, listener);
}
/**
* Read the large CSV file, default skip head line
* @param filePath read file path
* @param cls
* @param charset
* @param context Can init CsvWriter or CsvReader
* @param listener Synchronous call per read line
* @param
*/
public static void readOnListener(String filePath, Class cls,
Charset charset,
CsvContext context,
CsvListener listener) throws Exception {
final CsvReader reader = CsvFactory.getReader(filePath, charset);
readOnListenerAndWithContext(reader, cls, DEFAULT_SKIP_ROW, context, listener);
}
private static void readOnListenerAndWithContext(CsvReader reader, Class cls,
int skipRow,
CsvContext context,
CsvListener listener) {
try {
for (int i = 0; i < skipRow; i++) {
reader.readRecord();
}
CsvDataBuilder builder = new CsvDataBuilder(context);
builder.readWithListener(reader, cls, listener);
} catch (Exception e) {
throw new CsvUtilException(e);
} finally {
if (reader != null) {
reader.close();
}
}
}
private static List readContext(CsvReader reader,
Class cls, int skipRow, CsvContext context) {
try {
for (int i = 0; i < skipRow; i++) {
reader.readRecord();
}
CsvDataBuilder builder = new CsvDataBuilder(context);
return builder.read(reader, cls);
} catch (Exception e) {
throw new CsvUtilException(e);
} finally {
if (reader != null) {
reader.close();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy