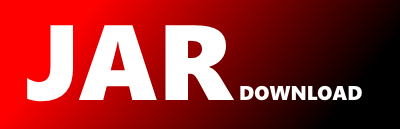
com.telewave.logger.aop.BusinessDataLoggerAop Maven / Gradle / Ivy
The newest version!
package com.telewave.logger.aop;
import java.io.IOException;
import java.net.URLDecoder;
import java.util.Date;
import java.util.UUID;
import javax.servlet.http.HttpServletRequest;
import com.telewave.logger.common.ResponseVO;
import com.telewave.logger.common.Utils;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.AfterReturning;
import org.aspectj.lang.annotation.AfterThrowing;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.annotation.Pointcut;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.env.Environment;
import org.springframework.stereotype.Component;
import org.springframework.util.StringUtils;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.telewave.logger.controller.BusinessDataLogController;
import com.telewave.logger.entity.BusinessDataLog;
import com.telewave.logger.entity.OperatorInfo;
@Aspect
@Component
public class BusinessDataLoggerAop {
private static final Logger logger = LoggerFactory.getLogger(BusinessDataLoggerAop.class);
@Autowired
BusinessDataLogController businessDataLogController;
@Autowired
private Environment env;
@Pointcut("@annotation(com.telewave.logger.aop.BusinessDataLogger)")
public void businessDataLog() {
System.out.println("Before-------------------");
}
@Before("businessDataLog()&&" + "@annotation(businessDataLogger)")
public void deBefore(JoinPoint joinPoint, BusinessDataLogger businessDataLogger) throws Throwable {
// 接收到请求,记录请求内容
String fullName = joinPoint.getTarget().getClass().getName() + "." + joinPoint.getSignature().getName();
try{
ServletRequestAttributes attributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
HttpServletRequest request = attributes.getRequest();
// 获取软件信息
if(env.getProperty("app.name")==null || env.getProperty("app.version")==null){
Utils.TWLOGGER_BUSINESSDATALOG.info(String.format("执行%s时,软件业务日志上报获取软件信息失败!软件信息为空!", fullName));
return;
}
//操作者获取
OperatorInfo operatorInfo = null;
String strOperatorInfo = request.getHeader("operator-info");
if (! StringUtils.isEmpty(strOperatorInfo)) {
String result = URLDecoder.decode(strOperatorInfo, "UTF-8");
if (!Utils.isJSONValid(result)) {
Utils.TWLOGGER_BUSINESSDATALOG.info(String.format("执行%s时,软件业务日志上报获取operator-info对象【%s】不合法!", fullName,result));
}else {
operatorInfo = JSON.parseObject(result, OperatorInfo.class);
}
}
String businessdata = "";
if (!StringUtils.isEmpty(joinPoint.getArgs()[0])) {
businessdata = JSONObject.toJSON(joinPoint.getArgs()[0]).toString();
}else{
Utils.TWLOGGER_BUSINESSDATALOG.info("执行%s时,软件业务日志上报失败,参数为空!", fullName);
return;
}
Date dt = new Date();
int editType = businessDataLogger.editType()[0].getIndex();
String keyNames[] = businessDataLogger.primaryKeyName().split(",");
// 警情数据变更分单条插入或批量插入
if(businessDataLogger.paramType()[0].getIndex() == 0){
//警情数据变更单条插入时
BusinessDataLog businessDataLog = new BusinessDataLog();
// 获取软件信息
businessDataLog.setSoftware(env.getProperty("app.name")); //软件名称
businessDataLog.setVersion(env.getProperty("app.version")); //软件版本号
if (operatorInfo != null) {
businessDataLog.setOperatorid(operatorInfo.getId()); // 操作者ID
businessDataLog.setOperator(operatorInfo.getLoginName()); // 操作者登录名
businessDataLog.setOperatordeptcode(operatorInfo.getOrganId());// 操作者机构ID
businessDataLog.setOperatordeptname(operatorInfo.getOrganName());// 操作者机构名称
businessDataLog.setSeatno(operatorInfo.getSeatNo());// 操作者坐席
}
String primaryKeyValue = "";
if(editType == 1 || editType == 2){
// 当为插入或更新操作时,记录实体json,并获取主键值
JSONObject jsonObject = JSON.parseObject(businessdata);
// 兼容多主键情况
for(int i=0; i responseVO = businessDataLogController.insert(businessDataLog);
if(responseVO!=null && responseVO.getData()){
Utils.TWLOGGER_BUSINESSDATALOG.info(String.format("执行%s时,软件业务操作日志上报成功!", fullName));
}else{
Utils.TWLOGGER_BUSINESSDATALOG.info(String.format("执行%s时,软件业务操作日志上报失败!", fullName));
}
}else{
//警情数据变更批量插入时
if(businessdata != null && businessdata.startsWith("[") && businessdata.endsWith("]")){
JSONArray jsonArray = JSONArray.parseArray(businessdata);
for(int j=0; j responseVO = businessDataLogController.insert(businessDataLog);
if(responseVO!=null && responseVO.getData()){
Utils.TWLOGGER_BUSINESSDATALOG.info(String.format("执行%s时,软件业务操作日志上报成功!", fullName));
}else{
Utils.TWLOGGER_BUSINESSDATALOG.info(String.format("执行%s时,软件业务操作日志上报失败!", fullName));
}
}
}
}
}catch (Exception e){
Utils.TWLOGGER_BUSINESSDATALOG.info(String.format("执行%s时,软件业务操作日志异常失败!", fullName));
Utils.saveLoggerToFileError(e);
}
}
// 环绕通知,环绕增强,相当于MethodInterceptor
/* @Around("businessDataLog()")
public Object arround(ProceedingJoinPoint pjp) {
try {
Object o = pjp.proceed();
return o;
} catch (Throwable e) {
e.printStackTrace();
return null;
}
}*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy