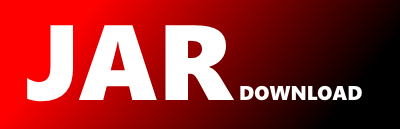
com.telewave.logger.entity.BusinessDataLog Maven / Gradle / Ivy
The newest version!
package com.telewave.logger.entity;
import com.telewave.logger.aop.TwLoggerField;
import java.util.Date;
public class BusinessDataLog {
@TwLoggerField(allowNull = false)
private String id; //主键 UUID
@TwLoggerField(allowNull = false)
private String software; //软件名称
@TwLoggerField(allowNull = false)
private String version; //软件版本
@TwLoggerField(allowNull = false,dateFormat = "yyyy-MM-dd HH:mm:ss")
private Date createlogdate; //日志创建时间
@TwLoggerField(allowNull = false)
private String clientip; //日志来源IP
@TwLoggerField(allowNull = false)
private Integer edittype; //编辑类别(0--Query,1--Insert,2--Update,3--Delete)
private String tablename; //表名
private String primarykeyvalue; //主键
private String seatno; //坐席号
private String operator; //操作者名称
private String operatorid; //操作者id
private String operatordeptname; //操作者所属机构名称
private String operatordeptcode; //操作者所属机构编码
private Date createdate; //入库时间
private int reportflag; //上报标记:-1为新数据,0为待上报,1为已上报
private String reportcode; //上报失败返回状态。
@TwLoggerField(allowNull = false)
private String businessdata; //警情及相关数据 JSon
public String getId() {
return id;
}
public void setId(String id) {
this.id = id == null ? null : id.trim();
}
public String getSoftware() {
return software;
}
public void setSoftware(String software) {
this.software = software == null ? null : software.trim();
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version == null ? null : version.trim();
}
public Date getCreatelogdate() {
return createlogdate;
}
public void setCreatelogdate(Date createlogdate) {
this.createlogdate = createlogdate;
}
public String getClientip() {
return clientip;
}
public void setClientip(String clientip) {
this.clientip = clientip == null ? null : clientip.trim();
}
public Integer getEdittype() {
return edittype;
}
public void setEdittype(Integer edittype) {
this.edittype = edittype;
}
public String getTablename() {
return tablename;
}
public void setTablename(String tablename) {
this.tablename = tablename == null ? null : tablename.trim();
}
public String getPrimarykeyvalue() {
return primarykeyvalue;
}
public void setPrimarykeyvalue(String primarykeyvalue) {
this.primarykeyvalue = primarykeyvalue == null ? null : primarykeyvalue.trim();
}
public String getSeatno() {
return seatno;
}
public void setSeatno(String seatno) {
this.seatno = seatno == null ? null : seatno.trim();
}
public String getOperator() {
return operator;
}
public void setOperator(String operator) {
this.operator = operator == null ? null : operator.trim();
}
public String getOperatorid() {
return operatorid;
}
public void setOperatorid(String operatorid) {
this.operatorid = operatorid == null ? null : operatorid.trim();
}
public String getOperatordeptname() {
return operatordeptname;
}
public void setOperatordeptname(String operatordeptname) {
this.operatordeptname = operatordeptname == null ? null : operatordeptname.trim();
}
public String getOperatordeptcode() {
return operatordeptcode;
}
public void setOperatordeptcode(String operatordeptcode) {
this.operatordeptcode = operatordeptcode == null ? null : operatordeptcode.trim();
}
public Date getCreatedate() {
return createdate;
}
public void setCreatedate(Date createdate) {
this.createdate = createdate;
}
public String getBusinessdata() {
return businessdata;
}
public void setBusinessdata(String businessdata) {
this.businessdata = businessdata == null ? null : businessdata.trim();
}
public int getReportflag() {
return reportflag;
}
public void setReportflag(int reportflag) {
this.reportflag = reportflag;
}
public String getReportcode() {
return reportcode;
}
public void setReportcode(String reportcode) {
this.reportcode = reportcode;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy