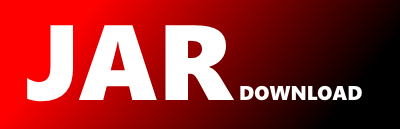
ix.IxWindowSkip Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ixjava Show documentation
Show all versions of ixjava Show documentation
ixjava developed by David Karnok
/*
* Copyright 2011-2016 David Karnok
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ix;
import java.util.*;
final class IxWindowSkip extends IxSource> {
final int size;
final int skip;
static final Object NULL = new Object();
IxWindowSkip(Iterable source, int size, int skip) {
super(source);
this.size = size;
this.skip = skip;
}
@Override
public Iterator> iterator() {
return new WindowIterator(source.iterator(), size, skip);
}
static final class WindowIterator extends IxSourceIterator> {
final int size;
int index;
final int skip;
WindowIterable current;
WindowIterator(Iterator it, int size, int skip) {
super(it);
this.size = size;
this.skip = skip;
}
@Override
protected boolean moveNext() {
int i = index;
for (;;) {
if (!it.hasNext()) {
done = true;
return false;
}
T v = it.next();
if (i++ == 0) {
index = i;
WindowIterable c = new WindowIterable(this, size);
current = c;
c.iterator.offer(v);
value = c;
hasValue = true;
return true;
}
WindowInnerIterator ci = current.iterator;
if (ci.offered < size) {
ci.offer(v);
}
if (i == skip) {
i = 0;
}
}
}
boolean moveInner() {
if (!it.hasNext()) {
return false;
}
T v = it.next();
current.iterator.offer(v);
index++;
return true;
}
}
static final class WindowIterable extends Ix {
final WindowInnerIterator iterator;
boolean once;
WindowIterable(WindowIterator parent, int size) {
this.iterator = new WindowInnerIterator(parent, size);
}
@Override
public Iterator iterator() {
if (!once) {
once = true;
return iterator;
}
throw new IllegalStateException("This Window Ix iterable can be consumed only once.");
}
}
static final class WindowInnerIterator extends IxBaseIterator {
final WindowIterator parent;
final ArrayDeque
© 2015 - 2025 Weber Informatics LLC | Privacy Policy