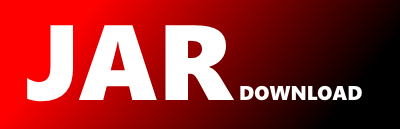
hu.akarnokd.reactive4java.util.Observables Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reactive4java Show documentation
Show all versions of reactive4java Show documentation
reactive4java developed by David Karnok
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy