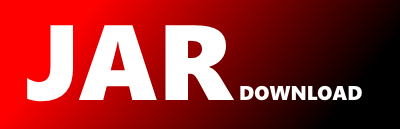
hu.akarnokd.rxjava2.internal.operators.OperatorMaterialize Maven / Gradle / Ivy
/**
* Copyright 2015 David Karnok and Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
* the License for the specific language governing permissions and limitations under the License.
*/
package hu.akarnokd.rxjava2.internal.operators;
import java.util.concurrent.atomic.*;
import org.reactivestreams.*;
import hu.akarnokd.rxjava2.*;
import hu.akarnokd.rxjava2.Observable.Operator;
import hu.akarnokd.rxjava2.internal.subscriptions.SubscriptionHelper;
import hu.akarnokd.rxjava2.internal.util.BackpressureHelper;
public enum OperatorMaterialize implements Operator>, Object> {
INSTANCE;
@SuppressWarnings({ "rawtypes", "unchecked" })
public static Operator>, T> instance() {
return (Operator)INSTANCE;
}
@Override
public Subscriber super Object> apply(Subscriber super Try>> t) {
return new MaterializeSubscriber
© 2015 - 2025 Weber Informatics LLC | Privacy Policy