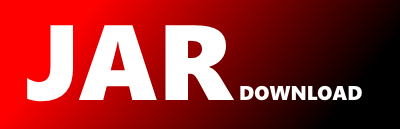
hu.akarnokd.rxjava2.subjects.PublishSubject Maven / Gradle / Ivy
Show all versions of rxjava2-backport Show documentation
/**
* Copyright 2015 David Karnok and Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
* the License for the specific language governing permissions and limitations under the License.
*/
package hu.akarnokd.rxjava2.subjects;
import java.util.concurrent.atomic.*;
import org.reactivestreams.*;
import hu.akarnokd.rxjava2.exceptions.MissingBackpressureException;
import hu.akarnokd.rxjava2.functions.IntFunction;
import hu.akarnokd.rxjava2.internal.subscriptions.SubscriptionHelper;
import hu.akarnokd.rxjava2.internal.util.*;
import hu.akarnokd.rxjava2.plugins.RxJavaPlugins;
/**
* A Subject that multicasts events to Subscribers that are currently subscribed to it.
*
* The subject does not coordinate backpressure for its subscribers and implements a weaker onSubscribe which
* calls requests Long.MAX_VALUE from the incoming Subscriptions. This makes it possible to subscribe the PublishSubject
* to multiple sources (note on serialization though) unlike the standard contract on Subscriber. Child subscribers, however, are not overflown but receive an
* IllegalStateException in case their requested amount is zero.
*
*
The implementation of onXXX methods are technically thread-safe but non-serialized calls
* to them may lead to undefined state in the currently subscribed Subscribers.
*
*
Due to the nature Observables are constructed, the PublishSubject can't be instantiated through
* {@code new} but must be created via the {@link #create()} method.
*
* @param the value type multicast to Subscribers.
*/
public final class PublishSubject extends Subject {
/**
* Constructs a PublishSubject.
* @param the value type
* @return the new PublishSubject
*/
public static PublishSubject create() {
State state = new State();
return new PublishSubject(state);
}
/** Holds the terminal event and manages the array of subscribers. */
final State state;
/**
* Indicates the subject has been terminated. It is checked in the onXXX methods in
* a relaxed matter: concurrent calls may not properly see it (which shouldn't happen if
* the reactive-streams contract is held).
*/
boolean done;
protected PublishSubject(State state) {
super(state);
this.state = state;
}
@Override
public void onSubscribe(Subscription s) {
if (done) {
s.cancel();
return;
}
// PublishSubject doesn't bother with request coordination.
s.request(Long.MAX_VALUE);
}
@Override
public void onNext(T t) {
if (done) {
return;
}
if (t == null) {
onError(new NullPointerException());
return;
}
for (PublishSubscriber s : state.subscribers()) {
s.onNext(t);
}
}
@Override
public void onError(Throwable t) {
if (done) {
RxJavaPlugins.onError(t);
return;
}
done = true;
if (t == null) {
t = new NullPointerException();
}
for (PublishSubscriber s : state.terminate(t)) {
s.onError(t);
}
}
@Override
public void onComplete() {
if (done) {
return;
}
done = true;
for (PublishSubscriber s : state.terminate()) {
s.onComplete();
}
}
@Override
public boolean hasSubscribers() {
return state.subscribers().length != 0;
}
@Override
public boolean hasValue() {
return false;
}
@Override
public T getValue() {
return null;
}
@Override
public T[] getValues(T[] array) {
if (array.length != 0) {
array[0] = null;
}
return array;
}
@Override
public Throwable getThrowable() {
Object o = state.get();
if (o == State.COMPLETE) {
return null;
}
return (Throwable)o;
}
@Override
public boolean hasThrowable() {
Object o = state.get();
return o != null && o != State.COMPLETE;
}
@Override
public boolean hasComplete() {
return state.get() == State.COMPLETE;
}
/**
* Contains the state of the Subject, including the currently subscribed clients and the
* terminal value.
*
* @param the value type of the events
*/
@SuppressWarnings("rawtypes")
static final class State extends AtomicReference