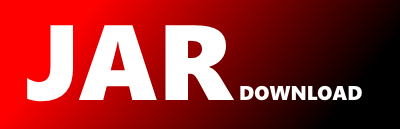
hu.akarnokd.rxjava2.subjects.nbp.NbpAsyncSubject Maven / Gradle / Ivy
Show all versions of rxjava2-backport Show documentation
/**
* Copyright 2015 David Karnok and Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
* the License for the specific language governing permissions and limitations under the License.
*/
package hu.akarnokd.rxjava2.subjects.nbp;
import java.lang.reflect.Array;
import java.util.concurrent.atomic.*;
import hu.akarnokd.rxjava2.disposables.*;
import hu.akarnokd.rxjava2.internal.util.NotificationLite;
import hu.akarnokd.rxjava2.plugins.RxJavaPlugins;
/**
* An NbpSubject that emits the very last value followed by a completion event or the received error to NbpSubscribers.
*
* The implementation of onXXX methods are technically thread-safe but non-serialized calls
* to them may lead to undefined state in the currently subscribed NbpSubscribers.
*
*
Due to the nature Observables are constructed, the NbpAsyncSubject can't be instantiated through
* {@code new} but must be created via the {@link #create()} method.
*
* @param the value type
*/
public final class NbpAsyncSubject extends NbpSubject {
public static NbpAsyncSubject create() {
State state = new State();
return new NbpAsyncSubject(state);
}
final State state;
protected NbpAsyncSubject(State state) {
super(state);
this.state = state;
}
@Override
public void onSubscribe(Disposable d) {
if (state.subscribers == State.TERMINATED) {
d.dispose();
}
}
@Override
public void onNext(T value) {
state.onNext(value);
}
@Override
public void onError(Throwable e) {
state.onError(e);
}
@Override
public void onComplete() {
state.onComplete();
}
@Override
public boolean hasSubscribers() {
return state.subscribers.length != 0;
}
@Override
public Throwable getThrowable() {
Object o = state.get();
if (NotificationLite.isError(o)) {
return NotificationLite.getError(o);
}
return null;
}
@Override
public T getValue() {
Object o = state.get();
if (o != null) {
if (NotificationLite.isComplete(o) || NotificationLite.isError(o)) {
return null;
}
return NotificationLite.getValue(o);
}
return null;
}
@SuppressWarnings("unchecked")
@Override
public T[] getValues(T[] array) {
Object o = state.get();
if (o != null) {
if (NotificationLite.isComplete(o) || NotificationLite.isError(o)) {
if (array.length != 0) {
array[0] = null;
}
} else {
T v = NotificationLite.getValue(o);
if (array.length != 0) {
array[0] = v;
if (array.length != 1) {
array[1] = null;
}
} else {
array = (T[])Array.newInstance(array.getClass().getComponentType(), 1);
array[0] = v;
}
}
} else {
if (array.length != 0) {
array[0] = null;
}
}
return array;
}
@Override
public boolean hasComplete() {
return state.subscribers == State.TERMINATED && !NotificationLite.isError(state.get());
}
@Override
public boolean hasThrowable() {
return NotificationLite.isError(state.get());
}
@Override
public boolean hasValue() {
Object o = state.get();
return o != null && !NotificationLite.isComplete(o) && !NotificationLite.isError(o);
}
static final class State extends AtomicReference