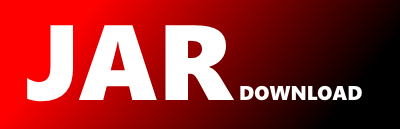
hu.akarnokd.rxjava2.subjects.nbp.NbpBehaviorSubject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rxjava2-backport Show documentation
Show all versions of rxjava2-backport Show documentation
rxjava2-backport developed by David Karnok
/**
* Copyright 2015 David Karnok and Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
* the License for the specific language governing permissions and limitations under the License.
*/
package hu.akarnokd.rxjava2.subjects.nbp;
import java.lang.reflect.Array;
import java.util.concurrent.atomic.*;
import java.util.concurrent.locks.*;
import hu.akarnokd.rxjava2.disposables.Disposable;
import hu.akarnokd.rxjava2.functions.Predicate;
import hu.akarnokd.rxjava2.internal.functions.Objects;
import hu.akarnokd.rxjava2.internal.util.*;
import hu.akarnokd.rxjava2.plugins.RxJavaPlugins;
public final class NbpBehaviorSubject extends NbpSubject {
public static NbpBehaviorSubject create() {
State state = new State();
return new NbpBehaviorSubject(state);
}
// TODO a plain create() would create a method ambiguity with Observable.create with javac
public static NbpBehaviorSubject createDefault(T defaultValue) {
Objects.requireNonNull(defaultValue, "defaultValue is null");
State state = new State();
state.lazySet(defaultValue);
return new NbpBehaviorSubject(state);
}
final State state;
protected NbpBehaviorSubject(State state) {
super(state);
this.state = state;
}
@Override
public void onSubscribe(Disposable s) {
state.onSubscribe(s);
}
@Override
public void onNext(T t) {
if (t == null) {
onError(new NullPointerException());
return;
}
state.onNext(t);
}
@Override
public void onError(Throwable t) {
if (t == null) {
t = new NullPointerException();
}
state.onError(t);
}
@Override
public void onComplete() {
state.onComplete();
}
@Override
public boolean hasSubscribers() {
return state.subscribers.length != 0;
}
/* test support*/ int subscriberCount() {
return state.subscribers.length;
}
@Override
public Throwable getThrowable() {
Object o = state.get();
if (NotificationLite.isError(o)) {
return NotificationLite.getError(o);
}
return null;
}
@Override
public T getValue() {
Object o = state.get();
if (NotificationLite.isComplete(o) || NotificationLite.isError(o)) {
return null;
}
return NotificationLite.getValue(o);
}
@Override
@SuppressWarnings("unchecked")
public T[] getValues(T[] array) {
Object o = state.get();
if (o == null || NotificationLite.isComplete(o) || NotificationLite.isError(o)) {
if (array.length != 0) {
array[0] = null;
}
return array;
}
T v = NotificationLite.getValue(o);
if (array.length != 0) {
array[0] = v;
if (array.length != 1) {
array[1] = null;
}
} else {
array = (T[])Array.newInstance(array.getClass().getComponentType(), 1);
array[0] = v;
}
return array;
}
@Override
public boolean hasComplete() {
Object o = state.get();
return NotificationLite.isComplete(o);
}
@Override
public boolean hasThrowable() {
Object o = state.get();
return NotificationLite.isError(o);
}
@Override
public boolean hasValue() {
Object o = state.get();
return o != null && !NotificationLite.isComplete(o) && !NotificationLite.isError(o);
}
static final class State extends AtomicReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy