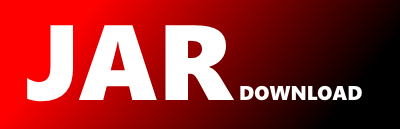
com.github.akurilov.commons.io.el.ExpressionInputBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-commons Show documentation
Show all versions of java-commons Show documentation
Common functionality Java library
package com.github.akurilov.commons.io.el;
import de.odysseus.el.util.SimpleContext;
import java.lang.reflect.Method;
import static com.github.akurilov.commons.io.el.ExpressionInput.ASYNC_MARKER;
import static com.github.akurilov.commons.io.el.ExpressionInput.FACTORY;
import static com.github.akurilov.commons.io.el.ExpressionInput.SYNC_MARKER;
public class ExpressionInputBuilder
implements ExpressionInput.Builder {
protected final SimpleContext ctx = new SimpleContext();
protected volatile String expr = null;
protected volatile T initial = null;
protected volatile Class type = null;
@Override
public final ExpressionInput.Builder expr(final String expr) {
this.expr = expr;
return this;
}
@Override
public final ExpressionInput.Builder initial(final T value) {
this.initial = value;
return this;
}
@Override
public final ExpressionInput.Builder type(final Class type) {
this.type = type;
return this;
}
@Override
public final ExpressionInput.Builder func(final String prefix, final String name, final Method method) {
ctx.setFunction(prefix, name, method);
return this;
}
@Override
public final ExpressionInput.Builder value(final String name, final Object val, final Class> type) {
final var ve = FACTORY.createValueExpression(val, type);
ctx.setVariable(name, ve);
return this;
}
@SuppressWarnings("unchecked")
@Override
public ExpressionInput build() {
if(null == expr) {
throw new NullPointerException("Expression shouldn't be null");
}
if(null == type) {
throw new NullPointerException("Type shouldn't be null");
}
if(expr.contains(SYNC_MARKER)) {
if(expr.contains(ASYNC_MARKER)) {
throw new IllegalArgumentException(
"The expression shouldn't contain both immediate ($) and deferred (#) expression markers"
);
}
return new SynchronousExpressionInputImpl<>(expr, initial, type, ctx);
} else {
return new ExpressionInputImpl<>(expr, initial, type, ctx);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy