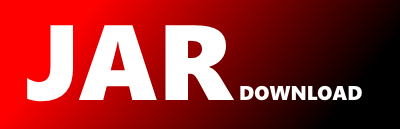
com.github.alex1304.ultimategdbot.api.database.GuildSettingsEntry Maven / Gradle / Ivy
package com.github.alex1304.ultimategdbot.api.database;
import java.lang.reflect.InvocationTargetException;
import java.util.Objects;
import java.util.function.BiConsumer;
import java.util.function.Function;
import org.hibernate.Session;
import com.github.alex1304.ultimategdbot.api.utils.DatabaseInputFunction;
import com.github.alex1304.ultimategdbot.api.utils.DatabaseOutputFunction;
import reactor.core.publisher.Mono;
/**
* Represents a guild configuration entry.
*
* @param - the guild settings entity type
* @param - the database value type
*/
public class GuildSettingsEntry {
private final Class entityClass;
private final Function valueGetter;
private final BiConsumer valueSetter;
private final DatabaseInputFunction stringToValue;
private final DatabaseOutputFunction valueToString;
public GuildSettingsEntry(Class entityClass, Function valueGetter, BiConsumer valueSetter,
DatabaseInputFunction stringToValue, DatabaseOutputFunction valueToString) {
this.entityClass = Objects.requireNonNull(entityClass);
this.valueGetter = Objects.requireNonNull(valueGetter);
this.valueSetter = Objects.requireNonNull(valueSetter);
this.stringToValue = Objects.requireNonNull(stringToValue);
this.valueToString = Objects.requireNonNull(valueToString);
}
public Class getEntityClass() {
return entityClass;
}
public D getRaw(Session s, long guildId) {
return valueGetter.apply(findOrCreate(s, guildId));
}
public void setRaw(Session s, D value, long guildId) {
var entity = findOrCreate(s, guildId);
valueSetter.accept(entity, value);
s.saveOrUpdate(entity);
}
public Mono getAsString(Session s, long guildId) {
return Mono.fromCallable(() -> getRaw(s, guildId))
.flatMap(raw -> valueToString.apply(raw, guildId))
.defaultIfEmpty("None");
}
public Mono setFromString(Session s, String strValue, long guildId) {
if (strValue == null) {
strValue = "None";
}
return stringToValue.apply(strValue, guildId)
.switchIfEmpty(Mono.fromRunnable(() -> setRaw(s, null, guildId)))
.flatMap(raw -> Mono.fromRunnable(() -> setRaw(s, raw, guildId)));
}
private E findOrCreate(Session s, long guildId) {
var entity = s.get(entityClass, guildId);
if (entity == null) {
try {
entity = entityClass.getConstructor().newInstance();
entity.setGuildId(guildId);
} catch (InstantiationException | IllegalAccessException | IllegalArgumentException
| InvocationTargetException | NoSuchMethodException | SecurityException e) {
throw new RuntimeException("Unable to create entity using reflection", e);
}
}
return entity;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy