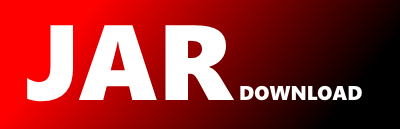
com.github.alex1304.ultimategdbot.api.utils.menu.ReactionToggleEvent Maven / Gradle / Ivy
package com.github.alex1304.ultimategdbot.api.utils.menu;
import java.util.Optional;
import java.util.function.Function;
import discord4j.core.event.domain.Event;
import discord4j.core.event.domain.message.ReactionAddEvent;
import discord4j.core.event.domain.message.ReactionRemoveEvent;
import discord4j.core.object.entity.Guild;
import discord4j.core.object.entity.Message;
import discord4j.core.object.entity.MessageChannel;
import discord4j.core.object.entity.User;
import discord4j.core.object.reaction.ReactionEmoji;
import discord4j.core.object.util.Snowflake;
import reactor.core.publisher.Mono;
public class ReactionToggleEvent {
private final Optional addEvent;
private final Optional removeEvent;
public ReactionToggleEvent(Event event) {
this.addEvent = Optional.of(event)
.filter(ReactionAddEvent.class::isInstance)
.map(ReactionAddEvent.class::cast);
this.removeEvent = Optional.of(event)
.filter(ReactionRemoveEvent.class::isInstance)
.map(ReactionRemoveEvent.class::cast);
if (addEvent.isEmpty() && removeEvent.isEmpty()) {
throw new IllegalArgumentException("Must be either ReactionAddEvent or ReactionRemoveEvent");
}
}
private T takeEither(Function fromAddEvent, Function fromRemoveEvent) {
return addEvent.map(fromAddEvent::apply)
.or(() -> removeEvent.map(fromRemoveEvent::apply))
.orElseThrow();
}
public Snowflake getUserId() {
return takeEither(ReactionAddEvent::getUserId, ReactionRemoveEvent::getUserId);
}
public Mono getUser() {
return takeEither(ReactionAddEvent::getUser, ReactionRemoveEvent::getUser);
}
public Snowflake getChannelId() {
return takeEither(ReactionAddEvent::getChannelId, ReactionRemoveEvent::getChannelId);
}
public Mono getChannel() {
return takeEither(ReactionAddEvent::getChannel, ReactionRemoveEvent::getChannel);
}
public Snowflake getMessageId() {
return takeEither(ReactionAddEvent::getMessageId, ReactionRemoveEvent::getMessageId);
}
public Mono getMessage() {
return takeEither(ReactionAddEvent::getMessage, ReactionRemoveEvent::getMessage);
}
public Optional getGuildId() {
return takeEither(ReactionAddEvent::getGuildId, ReactionRemoveEvent::getGuildId);
}
public Mono getGuild() {
return takeEither(ReactionAddEvent::getGuild, ReactionRemoveEvent::getGuild);
}
public ReactionEmoji getEmoji() {
return takeEither(ReactionAddEvent::getEmoji, ReactionRemoveEvent::getEmoji);
}
public boolean isAddEvent() {
return addEvent.isPresent();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy