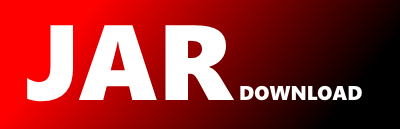
alexh.weak.BreadthChildIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dynamics Show documentation
Show all versions of dynamics Show documentation
Java library for handling nested dynamic data
package alexh.weak;
import java.util.Iterator;
import java.util.stream.Stream;
class BreadthChildIterator implements Iterator {
private final Dynamic root;
private int depth;
private Iterator current;
BreadthChildIterator(Dynamic root) {
this.root = root;
depth = 1;
current = root.children().iterator();
}
private Stream nextDepth() {
Stream childrenAtNextDepth = root.children();
int nextDepth = 1;
while (nextDepth <= this.depth) {
childrenAtNextDepth = childrenAtNextDepth.flatMap(Dynamic::children);
nextDepth += 1;
}
return childrenAtNextDepth;
}
private boolean moveDepthIfAvailable() {
Iterator nextDepth = nextDepth().iterator();
if (nextDepth.hasNext()) {
current = nextDepth;
depth += 1;
return true;
}
return false;
}
@Override
public boolean hasNext() {
return current.hasNext() || moveDepthIfAvailable();
}
@Override
public Dynamic next() {
if (!current.hasNext()) moveDepthIfAvailable();
return current.next();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy