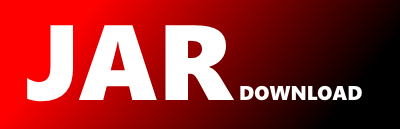
net.sf.weixinmp.saas.WeixinmpRequest Maven / Gradle / Ivy
The newest version!
package net.sf.weixinmp.saas;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.net.HttpURLConnection;
import java.net.URL;
import net.sf.weixinmp.ResponseErrorHandler;
import net.sf.weixinmp.ResponseHandler;
import net.sf.weixinmp.model.GenericObject;
import net.sf.weixinmp.model.deserializer.GenericObjectDeserializer;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.methods.HttpRequestBase;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.log4j.Logger;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public abstract class WeixinmpRequest {
private final static Logger LOGGER=Logger.getLogger(WeixinmpRequest.class);
protected static Gson gson=new Gson();
protected static final String RESTFUL_BASE="https://api.weixin.qq.com/cgi-bin";
protected ResponseErrorHandler errorHandler=new ResponseErrorHandler() {
public void handle(String errorCode, String errorMessage) {
LOGGER.error(errorCode+","+errorMessage);
}
};
protected ResponseHandler dataHandler=new ResponseHandler() {
public void handle(String jsonString) {
LOGGER.debug(jsonString);
}
};
public void setErrorHandler(ResponseErrorHandler errorHandler) {
this.errorHandler = errorHandler;
}
public void setDataHandler(ResponseHandler dataHandler) {
this.dataHandler = dataHandler;
}
public static GenericObject toNativeMap(String json) {
GsonBuilder gsonBuilder = new GsonBuilder();
gsonBuilder.registerTypeAdapter(GenericObject.class, new GenericObjectDeserializer());
return gsonBuilder.create().fromJson(json, GenericObject.class);
}
/**
* use java HttpURLConnection
*/
protected String requestContentStringURLConnection(String context, String method, String params ) {
StringBuffer bufferRes = new StringBuffer();
try {
URL realUrl = new URL(RESTFUL_BASE+context);
HttpURLConnection conn = (HttpURLConnection) realUrl.openConnection();
// 连接超时
conn.setConnectTimeout(25000);
// 读取超时 --服务器响应比较慢,增大时间
conn.setReadTimeout(25000);
HttpURLConnection.setFollowRedirects(true);
// 请求方式
conn.setRequestMethod(method);
conn.setDoOutput(true);
conn.setDoInput(true);
conn.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/45.0.2454.93 Safari/537.36 OPR/32.0.1948.69");
conn.setRequestProperty("Referer", "https://api.weixin.qq.com/");
conn.connect();
OutputStreamWriter out = new OutputStreamWriter(conn.getOutputStream());
//out.write(URLEncoder.encode(params,"UTF-8"));
out.write(params);
out.flush();
out.close();
InputStream in = conn.getInputStream();
BufferedReader read = new BufferedReader(new InputStreamReader(in,"UTF-8"));
String valueString = null;
while ((valueString=read.readLine())!=null){
bufferRes.append(valueString);
}
LOGGER.debug(bufferRes.toString());
in.close();
if (conn != null) {
// 关闭连接
conn.disconnect();
}
if(bufferRes.indexOf("errcode")!=-1){
if(errorHandler!=null) errorHandler.handle("50003", bufferRes.toString());
}
else {
if(dataHandler!=null) dataHandler.handle(bufferRes.toString());
}
} catch (Exception e) {
e.printStackTrace();
bufferRes.append(e.getLocalizedMessage());
if(errorHandler!=null) errorHandler.handle("50001", "internal error, "+e.getLocalizedMessage());
}
return bufferRes.toString();
}
/**
* use HttpClient
*/
protected String requestContentString(String context, String method, HttpEntity bodyEntity ) {
HttpClient privateClient = new DefaultHttpClient();
String ret ="";
try {
//TODO
//HttpHost proxyhost = new HttpHost("cn-proxy.jp.oracle.com", 80);
//privateClient.getParams().setParameter(ConnRoutePNames.DEFAULT_PROXY, proxyhost);
HttpRequestBase request = null;
if("GET".equalsIgnoreCase(method)){
request = new HttpGet(RESTFUL_BASE+context);
}
else if("POST".equalsIgnoreCase(method)){
request = new HttpPost(RESTFUL_BASE+context);
if(bodyEntity!=null){
((HttpPost)request).setEntity(bodyEntity);
}
}
//HttpGet get = new HttpGet(RESTFUL_BASE+context);
request.addHeader("Accept", "text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8");
request.addHeader("Accept-Encoding", "gzip, deflate");
request.addHeader("Cache-Control", "no-cache");
//request.addHeader("Connection", "keep-alive");
request.addHeader("Content-Type", "application/x-www-form-urlencoded");
request.addHeader("Accept-Language", "zh-CN,zh;q=0.8,en;q=0.6,id;q=0.4,zh-TW;q=0.2");
request.addHeader("Host", "www.nowcode.cn");
request.addHeader("Origin", "www.nowcode.cn");
request.addHeader("Pragma", "no-cache");
request.addHeader("Referer", "www.nowcode.cn");
request.addHeader("User-Agent", "Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/45.0.2454.93 Safari/537.36 OPR/32.0.1948.69");
HttpResponse response = privateClient.execute(request);
if(response.getStatusLine().getStatusCode()==200){
HttpEntity entity = response.getEntity();
InputStream in = entity.getContent();
//GZIPInputStream gzin = new GZIPInputStream(in);
StringBuilder result=new StringBuilder();
BufferedReader br = new BufferedReader(new InputStreamReader(in));
String temp = null;
while ((temp = br.readLine()) != null) {
result.append(temp);
}
br.close();
if(result.indexOf("errcode")!=-1){
if(errorHandler!=null) errorHandler.handle("50003", result.toString());
}
else {
if(dataHandler!=null) dataHandler.handle(result.toString());
ret = result.toString();
}
}
else {
if(errorHandler!=null) errorHandler.handle("50002", response.getStatusLine().toString());
}
} catch (Exception e) {
e.printStackTrace();
if(errorHandler!=null) errorHandler.handle("50001", "internal error, IO exception");
} finally{
privateClient.getConnectionManager().shutdown();
}
return ret;
}
protected String requestContentString(String context) {
return requestContentString(context, "GET", null);
}
protected String requestContentString(String context, String method) {
return requestContentString(context, method, null);
}
protected String requestContentString(String context, HttpEntity entity) {
return requestContentString(context, "POST", entity);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy