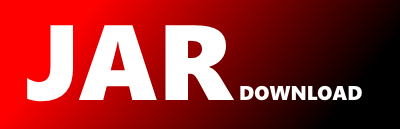
com.github.alittlehuang.data.query.support.model.CriteriaModel Maven / Gradle / Ivy
The newest version!
package com.github.alittlehuang.data.query.support.model;
import com.github.alittlehuang.data.query.specification.Criteria;
import com.github.alittlehuang.data.query.specification.WhereClause;
import lombok.Data;
import javax.persistence.LockModeType;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
@Data
public class CriteriaModel implements Criteria, Serializable {
protected final WhereClauseModel whereClause;
private final Class javaType;
private LockModeType lockModeType;
private Long offset;
private Long maxResults;
protected final List> selections = new ArrayList<>();
protected final List> groupings = new ArrayList<>();
protected final List> orders = new ArrayList<>();
protected final List> fetchAttributes = new ArrayList<>();
public CriteriaModel(WhereClause whereClause, Class type) {
this.whereClause = WhereClauseModel.convert(whereClause, type);
this.javaType = type;
}
public static CriteriaModel convert(Criteria criteria) {
if (criteria.getClass() == CriteriaModel.class) {
return (CriteriaModel) criteria;
}
Class javaType = criteria.getJavaType();
CriteriaModel result = new CriteriaModel<>(criteria.getWhereClause(), javaType);
result.getSelections().addAll(SelectionModel.convertSelection(criteria.getSelections(), javaType));
result.getGroupings().addAll(ExpressionModel.convertExpression(criteria.getGroupings(), javaType));
result.getOrders().addAll(OrdersModel.convertOrders(criteria.getOrders(), javaType));
result.getFetchAttributes().addAll(FetchAttributeModel.convertFetch(criteria.getFetchAttributes(), javaType));
result.setOffset(criteria.getOffset());
result.setMaxResults(criteria.getMaxResults());
result.setLockModeType(criteria.getLockModeType());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy