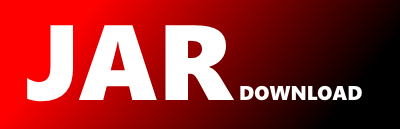
com.almasb.fxgl.entity.EntityGroup.kt Maven / Gradle / Ivy
/*
* FXGL - JavaFX Game Library. The MIT License (MIT).
* Copyright (c) AlmasB ([email protected]).
* See LICENSE for details.
*/
package com.almasb.fxgl.entity
import com.almasb.fxgl.core.Disposable
import java.util.function.Consumer
/**
* A group of entities of particular types.
* The group always contains active entities and listens
* for changes in the game world.
*
* @author Almas Baimagambetov ([email protected])
*/
class EntityGroup(
private val world: GameWorld,
initialEntities: List,
vararg entityTypes: Enum<*>
) : EntityWorldListener, Disposable {
private val types: List> = entityTypes.toList()
private val entities = ArrayList(initialEntities)
private val addList = ArrayList()
private val removeList = ArrayList()
/**
* @return shallow copy of the entities list (new list)
*/
val entitiesCopy: List
get(): List {
update()
return entities.filter { it.isActive }
}
init {
world.addWorldListener(this)
}
fun forEach(action: (Entity) -> Unit) {
entitiesCopy.forEach(action)
}
fun forEach(action: Consumer) {
entitiesCopy.forEach(action)
}
private fun update() {
entities.addAll(addList)
entities.removeAll(removeList)
addList.clear()
removeList.clear()
}
@Suppress("UNCHECKED_CAST")
override fun onEntityAdded(entity: Entity) {
if (types.any { entity.isType(it) }) {
addList.add(entity)
}
}
@Suppress("UNCHECKED_CAST")
override fun onEntityRemoved(entity: Entity) {
if (types.any { entity.isType(it) }) {
removeList.add(entity)
}
}
override fun dispose() {
world.removeWorldListener(this)
entities.clear()
addList.clear()
removeList.clear()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy