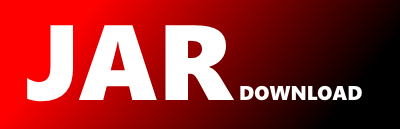
com.almasb.fxgl.particle.ParticleComponent.kt Maven / Gradle / Ivy
/*
* FXGL - JavaFX Game Library. The MIT License (MIT).
* Copyright (c) AlmasB ([email protected]).
* See LICENSE for details.
*/
package com.almasb.fxgl.particle
import com.almasb.fxgl.core.collection.UnorderedArray
import com.almasb.fxgl.core.pool.Pools
import com.almasb.fxgl.core.util.EmptyRunnable
import com.almasb.fxgl.entity.Entity
import com.almasb.fxgl.entity.component.Component
/**
* Allows adding particle effects to an entity.
*
* @author Almas Baimagambetov ([email protected])
*/
open class ParticleComponent(val emitter: ParticleEmitter) : Component() {
var onFinished: Runnable = EmptyRunnable
/**
* This is the entity whose view is used to render particles.
* Use of extra entity allows to render particles independently from
* the entity to which this component is attached. Otherwise, the entire
* set of emitted particles will be moved based on entity's view.
*/
val parent = Entity()
private val particles = UnorderedArray(256)
val isEmitterPaused: Boolean
get() = emitter.isPaused
override fun onUpdate(tpf: Double) {
if (parent.world == null) {
entity.world.addEntity(parent)
}
particles.addAll(emitter.emit(entity.x, entity.y))
val iter = particles.iterator()
while (iter.hasNext()) {
val p = iter.next()
if (p.update(tpf)) {
iter.remove()
parent.viewComponent.removeChild(p.view)
Pools.free(p)
} else {
if (p.view.parent == null)
parent.viewComponent.addChild(p.view)
}
}
if (particles.isEmpty && emitter.isFinished) {
onFinished.run()
}
}
fun pauseEmitter() {
emitter.pause()
}
fun resumeEmitter() {
emitter.resume()
}
override fun onRemoved() {
particles.forEach { Pools.free(it) }
particles.clear()
parent.removeFromWorld()
}
override fun isComponentInjectionRequired(): Boolean = false
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy