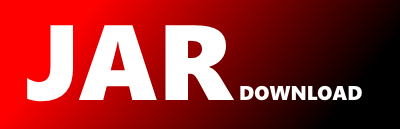
com.almasb.fxgl.pathfinding.astar.AStarGrid Maven / Gradle / Ivy
/*
* FXGL - JavaFX Game Library. The MIT License (MIT).
* Copyright (c) AlmasB ([email protected]).
* See LICENSE for details.
*/
package com.almasb.fxgl.pathfinding.astar;
import com.almasb.fxgl.core.collection.grid.Grid;
import com.almasb.fxgl.entity.Entity;
import com.almasb.fxgl.entity.GameWorld;
import com.almasb.fxgl.pathfinding.CellState;
import javafx.geometry.Rectangle2D;
import java.util.List;
import java.util.function.Function;
import java.util.stream.Collectors;
/**
* @author Almas Baimagambetov (AlmasB) ([email protected])
*/
public class AStarGrid extends Grid {
/**
* Constructs A* grid with A* cells using given width and height.
* All cells are initially {@link CellState#WALKABLE}.
*/
public AStarGrid(int width, int height) {
super(AStarCell.class, width, height, (x, y) -> new AStarCell(x, y, CellState.WALKABLE));
}
public List getWalkableCells() {
return getCells()
.stream()
.filter(c -> c.getState().isWalkable())
.collect(Collectors.toList());
}
/**
* Generates an A* grid from the given world data.
*
* @param world game world used to check for entities
* @param worldWidth in cell units (not pixels)
* @param worldHeight in cell units (not pixels)
* @param cellWidth in pixels
* @param cellHeight in pixels
* @param mapping maps entity types to WALKABLE / NOT_WALKABLE cells
*/
public static AStarGrid fromWorld(GameWorld world,
int worldWidth, int worldHeight,
int cellWidth, int cellHeight,
Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy