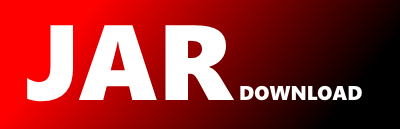
com.almondtools.util.collections.HashSets Maven / Gradle / Ivy
package com.almondtools.util.collections;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.Set;
public class HashSets {
private HashSet set;
private HashSets(boolean linked) {
if (linked) {
this.set = new LinkedHashSet();
} else {
this.set = new HashSet();
}
}
private HashSets(Collection extends T> set, boolean linked) {
if (linked) {
this.set = new LinkedHashSet(set);
} else {
this.set = new HashSet(set);
}
}
public static HashSets linked() {
return new HashSets(true);
}
public static HashSets linked(Collection set) {
return new HashSets(set, true);
}
public static HashSets hashed() {
return new HashSets(false);
}
public static HashSets hashed(Collection set) {
return new HashSets(set, false);
}
public static HashSets empty() {
return new HashSets(false);
}
public static HashSet of(T... elements) {
return new HashSet(Arrays.asList(elements));
}
public static HashSet of(Predicate cond, T... elements) {
HashSet list = new HashSet();
for (T element : elements) {
if (cond.evaluate(element)) {
list.add(element);
}
}
return list;
}
public static LinkedHashSet ofLinked(T... elements) {
return new LinkedHashSet(Arrays.asList(elements));
}
public static LinkedHashSet ofLinked(Predicate cond, T... elements) {
LinkedHashSet list = new LinkedHashSet();
for (T element : elements) {
if (cond.evaluate(element)) {
list.add(element);
}
}
return list;
}
public static HashSet intersectionOf(Set set, Set other) {
return new HashSets(set, false).intersect(other).build();
}
public static HashSet unionOf(Set set, Set other) {
return new HashSets(set, false).union(other).build();
}
public static HashSet complementOf(Set set, Set minus) {
return new HashSets(set, false).minus(minus).build();
}
public HashSets union(Set add) {
return addAll(add);
}
public HashSets add(T add) {
set.add(add);
return this;
}
public HashSets addConditional(boolean b, T add) {
if (b) {
set.add(add);
}
return this;
}
public HashSets addAll(Set add) {
set.addAll(add);
return this;
}
public HashSets addAll(T... add) {
set.addAll(Arrays.asList(add));
return this;
}
public HashSets minus(Set remove) {
return removeAll(remove);
}
public HashSets remove(T remove) {
set.remove(remove);
return this;
}
public HashSets removeConditional(boolean b, T remove) {
if (b) {
set.remove(remove);
}
return this;
}
public HashSets removeAll(Set remove) {
set.removeAll(remove);
return this;
}
public HashSets removeAll(T... remove) {
set.removeAll(Arrays.asList(remove));
return this;
}
public HashSets intersect(Set retain) {
return retainAll(retain);
}
public HashSets retain(T retain) {
Set retainAll = new HashSet();
retainAll.add(retain);
set.retainAll(retainAll);
return this;
}
public HashSets retainConditional(boolean b, T retain) {
if (b) {
Set retainAll = new HashSet();
retainAll.add(retain);
set.retainAll(retainAll);
}
return this;
}
public HashSets retainAll(Set retain) {
set.retainAll(retain);
return this;
}
public HashSets retainAll(T... retain) {
set.retainAll(Arrays.asList(retain));
return this;
}
public HashSet build() {
return set;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy