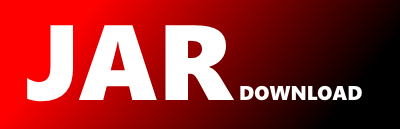
com.almondtools.util.collections.CollectionUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rexlex Show documentation
Show all versions of rexlex Show documentation
Regular expression matchers, searcher, lexers based on deterministic finite automata
package com.almondtools.util.collections;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
public class CollectionUtils {
public static char[] toCharArray(Collection charlist) {
char[] chars = new char[charlist.size()];
int i = 0;
for (Character c : charlist) {
chars[i] = c.charValue();
i++;
}
return chars;
}
public static List toIntegerList(int[] array) {
List list = new ArrayList(array.length);
for (int i : array) {
list.add(i);
}
return list;
}
public static List map(List list, ListMapping mapping) {
List result = new LinkedList();
for (F item : list) {
T mapped = mapping.map(item);
if (mapped != null) {
result.add(mapped);
}
}
return result;
}
public static Map map(Map map, MapMapping mapping) {
Map result = new LinkedHashMap();
for (Map.Entry item : map.entrySet()) {
TK mappedkey = mapping.key(item.getKey(), item.getValue());
TV mappedvalue = mapping.value(item.getKey(), item.getValue());
if (mappedkey != null && mappedvalue != null) {
result.put(mappedkey, mappedvalue);
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy